Advanced Web App AngularJS Techniques
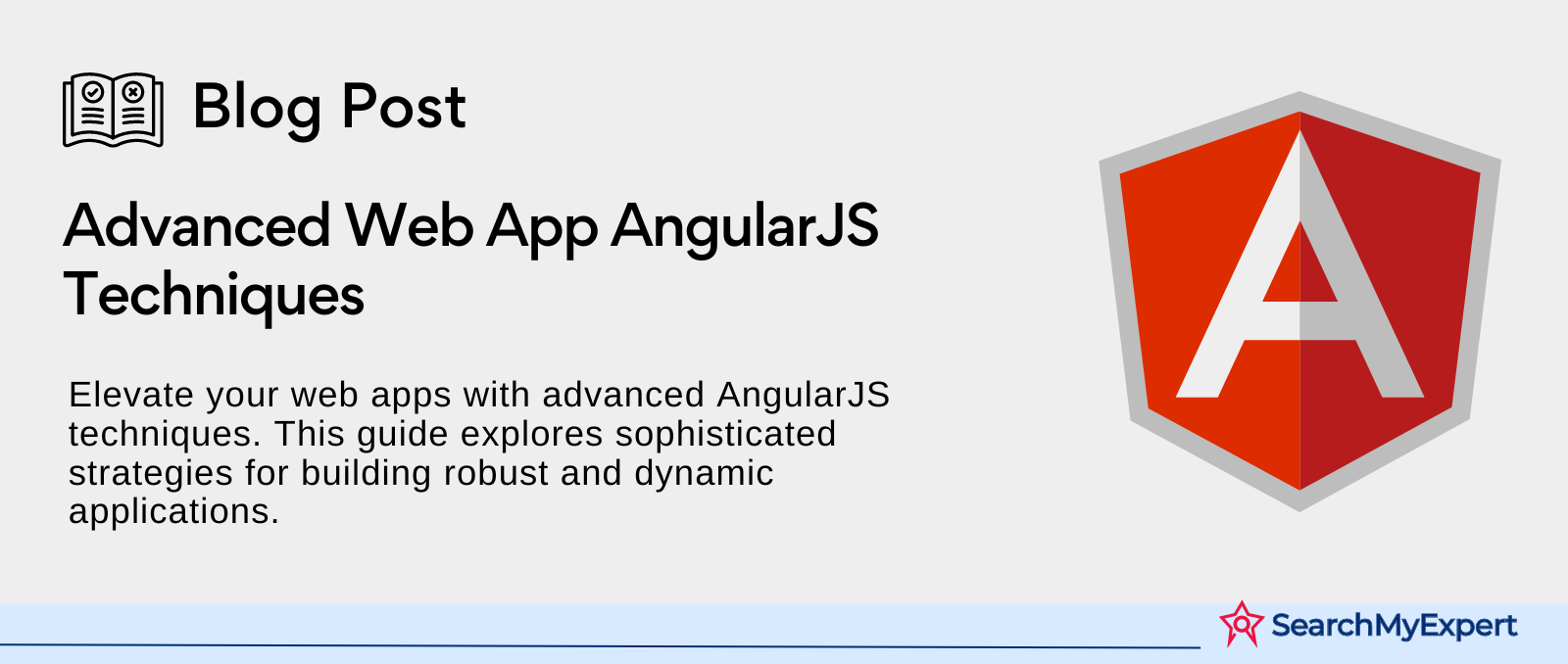
Introduction to AngularJS Controllers
AngularJS is a powerful framework designed to build dynamic web applications. Controllers are a fundamental aspect of AngularJS, providing the necessary mechanisms to connect data to the user interfaces. They act as an intermediary between models and views, handling the data transformation and logic required to present the user with a responsive and interactive experience. Below, we'll dive into the specifics of what controllers are, their role within the AngularJS framework, and their key responsibilities.
What are Controllers?
Controllers in AngularJS are defined JavaScript functions that are used to build up the application's data model. The controller's main job is to control the data flow between the model and the view. It is defined within an AngularJS module and can be attached to the DOM in the view layer through the ng-controller directive. Controllers manage the application logic and are responsible for responding to events initiated by the user and updating the model, which in turn updates the view.
Role in the AngularJS Framework
The role of controllers in AngularJS is critical for the following reasons:
- Model Initialization: Controllers initialize the scope with data and variables. This data is then used to render the view with dynamic information.
- UI Logic: They contain the logic for the UI, determining what happens when a user interacts with the interface, such as clicking a button or submitting a form.
- Separation of Concerns: Controllers help in maintaining a clean separation of concerns within the application. By separating the presentation layer from the business logic, AngularJS allows for more manageable and modular code.
Key Responsibilities of Controllers
Initialization of the Model
Controllers are responsible for setting up the initial state of the $scope object, which is crucial for data binding between the view and the model. This often involves fetching data from a server or initializing variables with default values.
Adding Behavior
Controllers add behavior to the scope object that can be invoked from the view. This includes functions and methods that execute when a user interacts with the application, facilitating a dynamic and interactive user experience.
Watching for Changes
Through the $scope object, controllers can watch for changes in the model's state or in form inputs. This allows the application to react dynamically to user input or changes in the data model, ensuring the UI stays up-to-date with the underlying data.
Business Logic
The business logic of the application is often encapsulated within controllers. This includes validation, computation, and data manipulation tasks that are necessary to support the application's core functions.
Dependency Injection
AngularJS supports dependency injection, allowing controllers to access other services and components within the application. This is used to modularize and reuse code, such as services for making HTTP requests or utilities for date formatting.
Defining and Creating Controllers in AngularJS
Defining and creating controllers is a crucial step in AngularJS application development, enabling the logical handling of data and user interactions. This step involves understanding the syntax for defining controllers, how to inject dependencies, and the controller lifecycle within the AngularJS framework.
Syntax for Defining a Controller Function
In AngularJS, controllers are defined using the .controller method of an AngularJS module. The basic syntax involves specifying the controller name and a constructor function. The constructor function is where you define the behavior of the controller, including initializing the model and defining functions for user interactions. While specific code examples are not provided here, it's important to note that this method allows you to encapsulate the controller's logic cleanly and modularly.
Injecting Dependencies Using the $scope Object
Dependency injection is a core feature of AngularJS, allowing controllers to access other AngularJS services and components. The $scope object is one of the most common dependencies injected into controllers. It acts as a glue between the controller and the view, providing a context where the model data is stored and manipulated. Other services, such as $http for AJAX calls, $timeout for delayed functions, and custom services, can also be injected into controllers to provide the necessary functionality. AngularJS uses the constructor function's arguments to determine which services to inject, making it straightforward to add the services your controller needs to interact with the rest of the application.
Understanding the Controller Lifecycle
The lifecycle of a controller in AngularJS starts when the AngularJS framework instantiates it during the application bootstrap process or when the corresponding view is rendered. The controller remains active until the view it is associated with is destroyed or the application navigates away from the view, leading to the controller's destruction. During its lifecycle, a controller can react to events, watch expressions for changes, and communicate with other components through the scope and events. Understanding this lifecycle is key to managing resources effectively, such as setting up and tearing down watchers or handling events.
Key Points in the Controller Lifecycle:
- Initialization: Upon creation, the controller initializes the model's state and binds functions to the $scope object or this context, preparing the view for interaction.
- Watching Data: Controllers can watch for changes in the data model or $scope variables, allowing them to react to user input or changes in application state dynamically.
- Clean-up: When a controller's lifecycle ends, it's important to release resources, such as event listeners or timeouts, to prevent memory leaks. AngularJS handles much of this automatically, but understanding the lifecycle helps in managing custom clean-up tasks efficiently.
Working with the $scope Object in AngularJS
The $scope object in AngularJS is a pivotal component that plays a crucial role in the interaction between the controller and the view. Understanding how to work with $scope is essential for effective AngularJS application development. This involves comprehending the scope hierarchy and inheritance, how to expose data and methods to the view, and implementing two-way data binding with the ng-model.
Scope Hierarchy and Inheritance
In AngularJS, scopes form a hierarchy that mirrors the DOM (Document Object Model) structure where the application is defined. This hierarchical structure allows for modular development and data encapsulation within different parts of the application.
- Root Scope: At the top of the hierarchy is the $rootScope, which is accessible by all parts of the application, making it a good place for data and methods that need to be globally available.
- Child Scopes: Each controller and directive can create its own child scope that inherits properties and methods from its parent scope, much like prototypical inheritance in JavaScript. This means that data and methods defined in a parent scope are accessible in child scopes, allowing for data sharing and communication between different parts of the application.
Exposing Data and Methods to the View
The $scope object is used to expose data and methods from the controller to the view. By attaching properties and functions to $scope, you make them accessible in the HTML template associated with the controller.
- Data Exposure: Data bound to $scope becomes available in the view, enabling dynamic rendering of content based on the model's state.
- Method Exposure: Functions defined on $scope can be called from the view, allowing for user interaction handling, such as responding to button clicks or form submissions.
Two-Way Data Binding with ng-model
One of the most powerful features of AngularJS is two-way data binding, particularly as implemented by the ng-model directive. This feature automatically synchronizes the data between the model (attached to $scope) and the view (input fields, select boxes, etc.).
- Real-Time Synchronization: When a user modifies an input field bound with ng-model, the corresponding model on $scope is immediately updated, and vice versa. This real-time synchronization eliminates the need for manual DOM manipulation or event handling to update the user interface in response to model changes.
- Form Inputs: ng-model is commonly used with form elements, such as <input>, <select>, and <textarea>, to implement forms that are dynamically bound to the underlying data model.
Controller Methods and Logic in AngularJS
In AngularJS, controllers are not just about defining and initializing the model; they are also crucial for implementing the logic that underpins the application's functionality. This includes performing actions and manipulating data, handling user interactions and events, and calling external services and APIs. Understanding how to effectively leverage controller methods and logic is key to building dynamic, efficient, and interactive AngularJS applications.
Performing Actions and Manipulating Data
Controllers in AngularJS are responsible for the business logic associated with the data model. This involves a variety of tasks, such as:
- Data Transformation: Manipulating and formatting data to be displayed or consumed by the user or an external service. This can include sorting lists, filtering datasets based on criteria, or aggregating information for summaries.
- Model Updates: Responding to changes within the application, controllers update the model, which in turn updates the view. This might involve adding, editing, or removing items from a list or updating individual data fields based on user input.
Handling User Interactions and Events
One of the primary roles of controllers is to handle interactions from the user, such as clicks, form submissions, and other events. This is achieved by:
- Event Listeners: Defining functions within the controller that are called in response to user actions. These functions can update the model, trigger animations, or validate input data, among other actions.
- $scope Methods: Through methods defined on $scope, the controller can respond to user interactions tied to specific elements in the view. This direct connection between the view and the model facilitates a responsive and interactive user experience.
Calling External Services and APIs
To extend the functionality of AngularJS applications, controllers often interact with external services and APIs for data retrieval, authentication, and other external operations. This involves:
- Using AngularJS Services: AngularJS provides built-in services like $http for making XMLHttpRequests to retrieve or send data to a server. Controllers can inject these services to interact with RESTful APIs, load external data, or submit data to a server.
- Custom Services: For more complex or reusable logic, developers can define their own services in AngularJS. These services can encapsulate API calls, data processing, or other tasks, and then be injected into any controller that requires them.
Best Practices for Controller Design in AngularJS
When developing applications with AngularJS, adhering to best practices in controller design can significantly enhance the maintainability, scalability, and efficiency of your code. Controllers play a crucial role in the AngularJS framework, acting as the intermediary between the view and the underlying business logic and data model. By following these guidelines, developers can ensure that their applications remain robust and easy to manage over time.
Separation of Concerns (Data, Logic, UI)
One of the foundational principles of good software architecture is the separation of concerns, which involves organizing code in a way that separates different aspects of the application:
- Data: The model representing the application's data should be distinct and decoupled from the user interface and the business logic.
- Logic: Business logic should reside in controllers or services, handling data processing, transformations, and decision-making processes without being tied directly to the UI.
- UI: The user interface, defined by views and directives in AngularJS, should focus solely on presenting data to the user and capturing user inputs, with minimal to no logic regarding data manipulation or business rules.
Keeping Controllers Focused and Lean
Controllers should be kept focused on their primary role: the interaction between the view and the model. This means avoiding the temptation to include too much logic or too many responsibilities within a single controller. Best practices include:
- Single Responsibility: Each controller should have a single responsibility and only manage a specific part of the view or a particular aspect of the application logic.
- Minimal Logic: Keep the amount of logic within controllers to a minimum. Heavy computations, data transformations, and complex business rules are better suited to services or utility functions.
Using Services for Complex Operations
For operations that involve complex logic, external API calls, or data manipulation, it's advisable to use AngularJS services. Services in AngularJS are singleton objects that can be injected into controllers (and other services) to perform specific tasks. This approach has several benefits:
- Reusability: Services can be reused across different parts of the application, avoiding duplication of code and ensuring consistency in the logic applied.
- Testability: Isolating complex operations within services makes it easier to write unit tests, as services can be tested independently of the controllers and views.
- Separation of Concerns: By delegating complex operations to services, controllers can remain focused on their primary role of linking the view with the model, further reinforcing the separation of concerns within the application.
Best Practices for Controller Design in AngularJS
When developing applications with AngularJS, adhering to best practices in controller design can significantly enhance the maintainability, scalability, and efficiency of your code. Controllers play a crucial role in the AngularJS framework, acting as the intermediary between the view and the underlying business logic and data model. By following these guidelines, developers can ensure that their applications remain robust and easy to manage over time.
Separation of Concerns (Data, Logic, UI)
One of the foundational principles of good software architecture is the separation of concerns, which involves organizing code in a way that separates different aspects of the application:
- Data: The model representing the application's data should be distinct and decoupled from the user interface and the business logic.
- Logic: Business logic should reside in controllers or services, handling data processing, transformations, and decision-making processes without being tied directly to the UI.
- UI: The user interface, defined by views and directives in AngularJS, should focus solely on presenting data to the user and capturing user inputs, with minimal to no logic regarding data manipulation or business rules.
Keeping Controllers Focused and Lean
Controllers should be kept focused on their primary role: the interaction between the view and the model. This means avoiding the temptation to include too much logic or too many responsibilities within a single controller. Best practices include:
- Single Responsibility: Each controller should have a single responsibility and only manage a specific part of the view or a particular aspect of the application logic.
- Minimal Logic: Keep the amount of logic within controllers to a minimum. Heavy computations, data transformations, and complex business rules are better suited to services or utility functions.
Using Services for Complex Operations
For operations that involve complex logic, external API calls, or data manipulation, it's advisable to use AngularJS services. Services in AngularJS are singleton objects that can be injected into controllers (and other services) to perform specific tasks. This approach has several benefits:
- Reusability: Services can be reused across different parts of the application, avoiding duplication of code and ensuring consistency in the logic applied.
- Testability: Isolating complex operations within services makes it easier to write unit tests, as services can be tested independently of the controllers and views.
- Separation of Concerns: By delegating complex operations to services, controllers can remain focused on their primary role of linking the view with the model, further reinforcing the separation of concerns within the application.
Common Controller Use Cases in AngularJS
Controllers in AngularJS are versatile components that handle a wide range of tasks related to user interaction, data manipulation, and application logic. Some of the most common use cases for controllers include managing CRUD operations, performing form validation and error handling, and implementing user authentication and authorization. Understanding these use cases is crucial for leveraging AngularJS effectively in web application development.
CRUD Operations (Create, Read, Update, Delete)
CRUD operations form the backbone of many web applications, enabling users to interact with data stored on a server or in a database. AngularJS controllers facilitate these operations as follows:
- Create: Controllers can process user input from forms to add new records to the data model, which can then be sent to a server via services.
- Read: They retrieve data from services, often making HTTP requests to a backend API, and then present this data in the view.
- Update: Controllers handle the modification of existing data, which involves capturing user input, updating the model, and sending the updated data back to the server.
- Delete: They process requests to remove data, updating the model and the view accordingly after the data is successfully deleted from the server.
Form Validation and Error Handling
Form validation is critical for ensuring that the data entered by users is correct and meaningful before it's processed or sent to a server. AngularJS provides powerful directives for form validation that can be easily integrated into controllers:
- Form Validation: Controllers can use AngularJS's built-in validation mechanisms to check user input for errors in real time, providing immediate feedback.
- Error Handling: They manage errors that may occur during data processing or server communication, displaying appropriate messages to the user and possibly rolling back any changes made to the model.
Implementing User Authentication and Authorization
User authentication and authorization are key aspects of securing web applications and ensuring that users can only access resources and perform actions according to their permissions:
- Authentication: Controllers can be used to manage the login process, handle user credentials, and communicate with backend services to verify user identity.
- Authorization: Once a user is authenticated, controllers can control access to various parts of the application based on the user's roles or permissions. This might involve conditionally showing or hiding parts of the UI and validating user actions against their allowed permissions.
Conclusion:
Mastering advanced controller techniques in AngularJS unlocks a new level of dynamic interaction and functionality for your web applications. By effectively using filters and directives alongside controllers, developers can create highly modular and reusable components, leading to cleaner and more maintainable code. Implementing custom directives allows for encapsulating complex UI behaviors, making your application more scalable and easier to understand. Furthermore, embracing unit testing for controllers ensures that your application remains robust and reliable as it evolves, catching potential issues early in the development cycle. Together, these advanced techniques provide the foundation for building sophisticated, efficient, and high-quality AngularJS applications that stand out in the modern web landscape.
Partner for success with elite
Angular JS Development Service Agencies.
share this page if you liked it 😊
Other Related Blogs
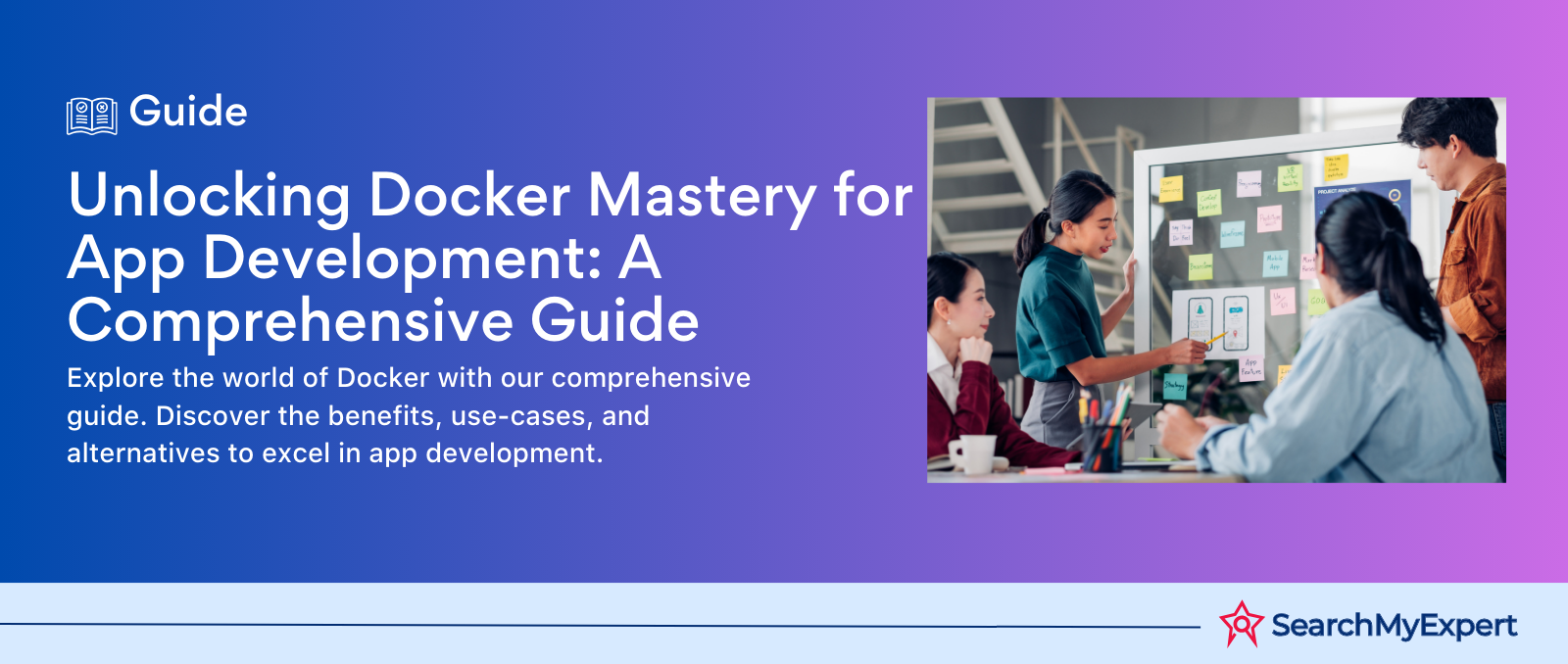
Mastering Docker for App Development: A Comprehensive Guide to Benefits, Use-Cases, and Alternatives
STAY UP TO DATE
GET PATH'S LATEST
Receive bi-weekly updates from the SME, and get a heads up on upcoming events.
Contact Us
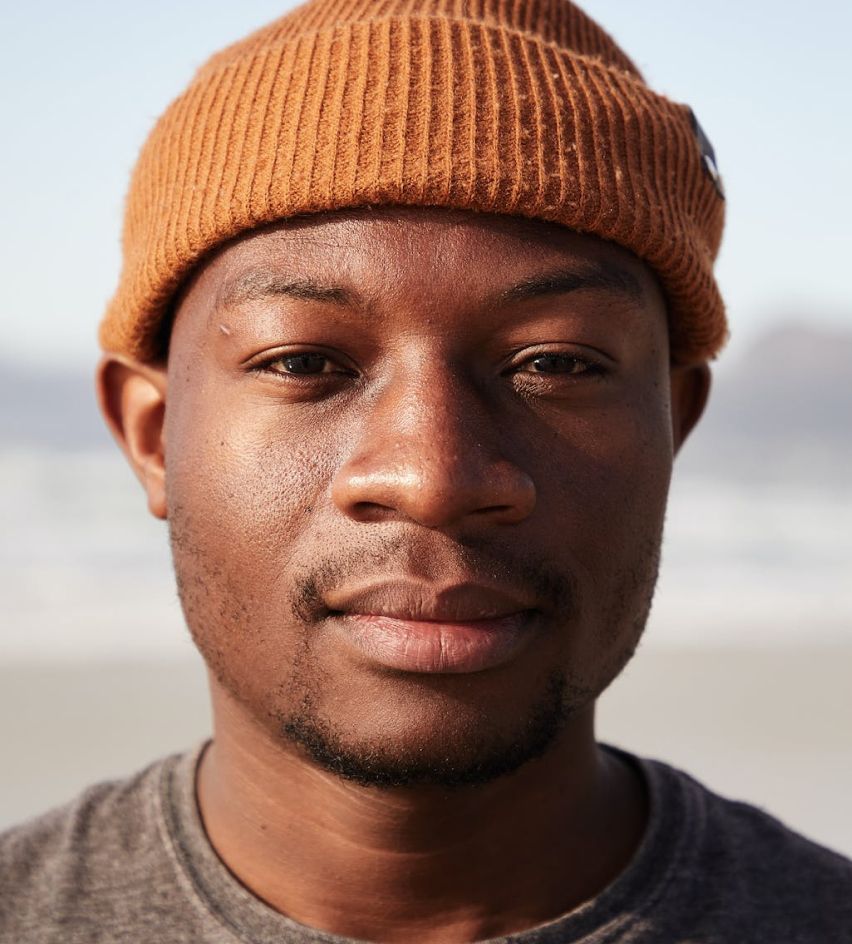
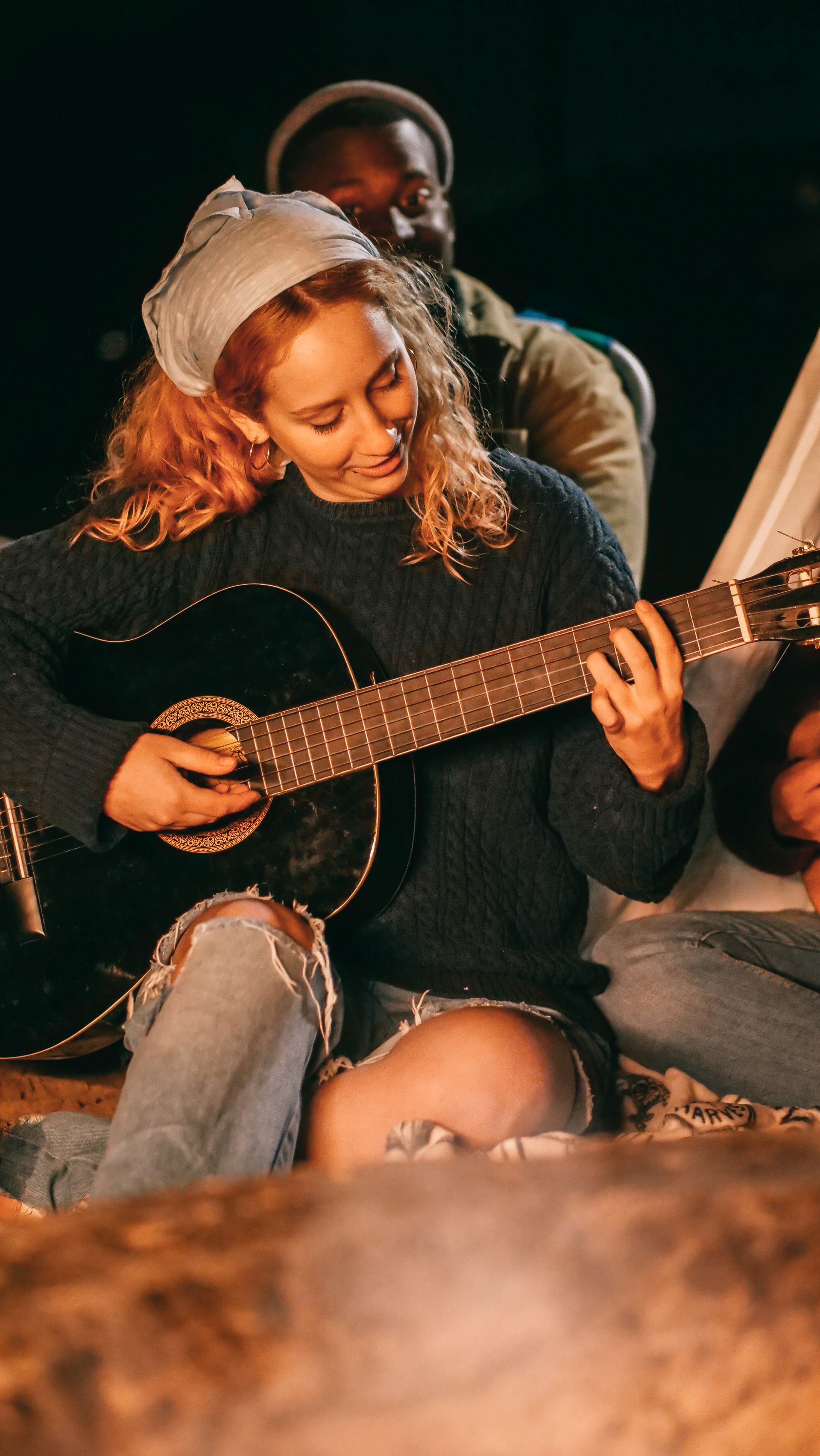