A Developer's Guide to Scaling AngularJS
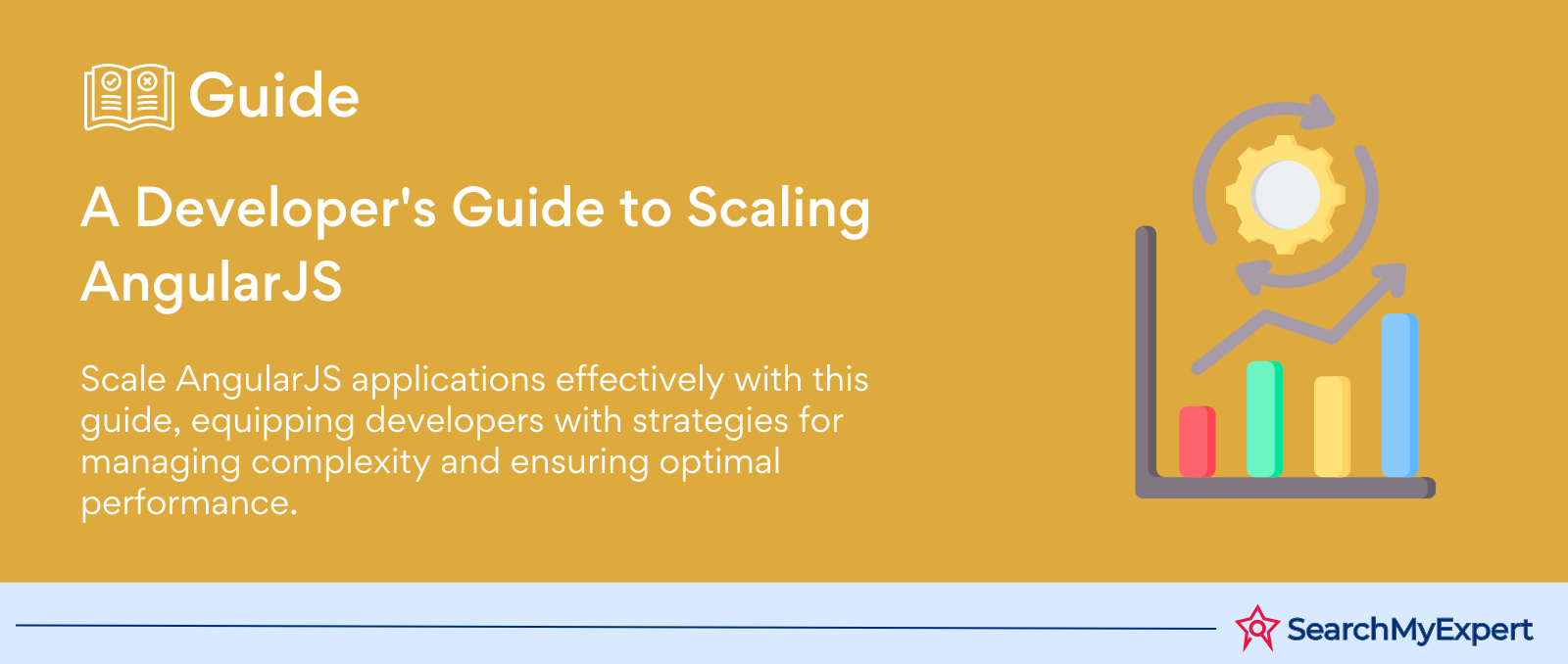
Scalability in AngularJS Web Applications: Navigating the Challenges
Scalability is a crucial aspect of modern web applications, acting as the backbone that supports their growth and ensures they can handle increasing loads with grace. It refers to the ability of an application to efficiently manage a growing number of requests without compromising on performance or user experience. In the context of web development, scalability is not just about handling traffic spikes; it's about building a foundation that supports expansion in users, data, and functionality over time.
The importance of scalability in web applications cannot be overstated. It is essential for maintaining fast response times, providing a seamless user experience, and ensuring reliability regardless of the number of concurrent users or the volume of data processed. As businesses grow and evolve, their web applications must be able to scale accordingly to accommodate new features, more users, and higher demands on resources.
The Unique Scalability Challenges of AngularJS Applications
AngularJS, a powerful framework maintained by Google, is widely used for building dynamic, single-page web applications (SPAs). It provides developers with a robust set of features to create interactive and responsive user interfaces. However, achieving scalability with AngularJS applications comes with its own set of challenges:
- Performance Bottlenecks: AngularJS's two-way data binding is a feature that stands out for its ability to synchronize the model and the view. While beneficial, this can also lead to performance issues in large applications if not managed carefully, as each binding adds to the watcher list that AngularJS needs to check, potentially leading to slower page rendering and updates.
- Large File Sizes: AngularJS applications can become quite large due to the inclusion of library code, application code, and templates. This increases the initial load time, which can negatively impact user experience and search engine ranking.
- Complexity in State Management: As applications grow, managing the state across components becomes increasingly complex. This can make it challenging to ensure that the application remains responsive and that data is consistently updated across the UI.
- Dependency Management: AngularJS applications often rely on numerous third-party libraries and modules. Ensuring that these dependencies are up-to-date and compatible with each other as the application scales can be a daunting task.
- Optimization for SEO: Single-page applications, like those built with AngularJS, face inherent challenges with search engine optimization (SEO). The dynamic rendering of content can make it difficult for search engine crawlers to index the application's content effectively.
Deep Dive into AngularJS Architecture: Core Components and Scalability
AngularJS, a structured framework for dynamic web applications, introduces a unique architecture that promotes modularity and testability. Understanding the core components of AngularJS applications is essential for developers looking to enhance scalability and performance. These components—modules, controllers, directives, and services—play pivotal roles in structuring applications. However, they also bring potential challenges that could impact scalability if not managed properly.
Core Components of AngularJS
Modules
Modules are the building blocks of AngularJS applications, encapsulating different parts of an app. They provide a container for the application's components, including controllers, services, directives, and filters. Modules make it easier to organize and manage code by separating functionalities, which is crucial for scalability. They enable developers to create reusable and maintainable code, reducing redundancy and facilitating easier updates as applications grow.
Controllers
Controllers are responsible for the business logic of AngularJS applications. They act as the intermediary between models and views, handling user input and updating the model and view accordingly. While controllers are essential for the MVC (Model-View-Controller) architecture, improper use, such as embedding too much logic or managing state within controllers, can lead to performance bottlenecks. For scalable AngularJS applications, it's vital to keep controllers lightweight and delegate complex tasks to services.
Directives
Directives are one of AngularJS's most powerful features, allowing developers to extend HTML with custom attributes and elements. They are used to create reusable components, manipulate the DOM, and build dynamic and interactive user interfaces. However, directives can also become a source of bottlenecks if they are overused or poorly implemented. Complex directives with extensive DOM manipulations can slow down the application, especially as the number of elements on the page grows.
Services
Services in AngularJS are singleton objects used to organize and share code across the application. They are ideal for performing common tasks such as data fetching, logging, and processing business logic. By centralizing code in services, applications become more modular, easier to test, and scalable. However, managing dependencies between services and ensuring they are efficiently used can be challenging as the application's complexity increases.
Optimizing AngularJS Applications: Enhancing Performance and Scalability
As AngularJS applications grow in complexity and size, optimizing performance becomes paramount. Efficiently structured code, optimized data binding, and judicious DOM manipulation are critical areas where thoughtful improvements can significantly impact application scalability. Additionally, leveraging caching mechanisms and performance profiling tools can offer substantial gains in responsiveness and user experience. Let's explore these optimization techniques in detail.
Optimizing Code Structure
A well-organized codebase is easier to maintain, debug, and scale. The following are strategies to optimize the code structure in AngularJS applications:
- Component-Based Architecture: Adopting a component-based architecture helps in breaking down the application into reusable and independent components, making the codebase more manageable as it grows.
- Lazy Loading: Implementing lazy loading for AngularJS modules can drastically reduce the initial load time by loading only the necessary parts of the application when they are required.
- Minification and Concatenation: Minifying and concatenating files can reduce the number of HTTP requests and the size of files that need to be loaded, thereby improving load times.
Enhancing Data Binding and DOM Manipulation
Data binding and DOM manipulation are areas where performance issues are commonly encountered in AngularJS applications. To optimize these:
- Limit Watchers: Reduce the number of watchers as much as possible since each adds to the digest cycle's complexity, impacting performance. Use one-way data binding (::) where updates from the model to the view are needed only once.
- Debounce ng-model Options: For inputs bound to models using ng-model, consider using the ng-model-options directive with a debounce setting. This can reduce the frequency of updates during typing, decreasing the load on the digest cycle.
- Use Virtual Scrolling: Implement virtual scrolling to render only a subset of a large list of items that are currently visible to the user. This can significantly reduce the number of DOM elements, improving rendering performance.
Leveraging Caching Mechanisms
Caching is an effective strategy to enhance performance, especially for applications that fetch data from APIs or have computationally expensive processes:
- HTTP Caching: Use HTTP caching headers to cache API responses where data does not change frequently. This reduces the load on the server and speeds up data retrieval.
- AngularJS CacheFactory: Utilize AngularJS's $cacheFactory service to cache data within the application. It can store and retrieve data efficiently, avoiding unnecessary computations or network requests.
Enhancing AngularJS Applications with Ecosystem Libraries
AngularJS's ecosystem is rich with libraries that extend its core functionalities, simplifying the development of complex applications. Libraries like ngRoute, ngResource, and UI-Router are especially pivotal for introducing modularity, efficient data handling, and sophisticated routing mechanisms. However, integrating third-party libraries comes with its set of considerations regarding application performance and scalability. Let's explore these libraries and the trade-offs involved.
ngRoute for Basic Routing
ngRoute is AngularJS's basic routing library, facilitating the mapping of URLs to corresponding views and controllers. It is straightforward to implement for smaller applications or those with simple routing needs.
- Advantages: Easy to use and set up, making it ideal for projects where complex routing is not required. It integrates seamlessly into AngularJS applications for basic navigation needs.
- Trade-offs: ngRoute is limited in functionality, lacking support for nested views or multiple-named views. This limitation can impact scalability and maintainability for more complex applications, potentially necessitating a switch to more advanced routing solutions as the application grows.
ngResource for RESTful Interaction
ngResource simplifies interactions with RESTful services by providing a higher-level abstraction on top of $http. It allows developers to interact with server-side data sources as objects, using actions that correspond to RESTful principles.
- Advantages: Reduces boilerplate code for CRUD operations and encourages the use of RESTful practices. It's particularly useful for applications that heavily interact with APIs, improving code readability and maintainability.
- Trade-offs: The abstraction provided by ngResource can introduce overhead for highly customized server interactions or when performance optimization is crucial. Developers might need to bypass these abstractions for fine-tuned control, potentially negating some of the benefits of using ngResource.
UI-Router for Advanced Routing
UI-Router offers a more powerful alternative to ngRoute, introducing concepts like nested views and multiple-named views. It provides a flexible routing framework that can handle complex routing needs, making it suitable for large-scale applications.
- Advantages: Supports advanced routing features, enhancing the modularity and navigational structure of AngularJS applications. Its ability to manage nested states and parameters makes it ideal for building sophisticated user interfaces.
- Trade-offs: The complexity of UI-Router can be a double-edged sword. While it offers greater flexibility, it also introduces a steeper learning curve and potentially higher initial development overhead. Additionally, improper use can lead to performance issues, especially in applications with deeply nested views or excessive watch expressions.
Enhancing AngularJS with Server-Side Rendering (SSR): A Path to Improved SEO and Performance
Server-side rendering (SSR) is a technique that significantly influences web application performance and search engine optimization (SEO). It involves generating the full HTML for a page on the server in response to a user's request, before sending it to the client. This approach contrasts with client-side rendering, where the browser downloads a minimal HTML page, retrieves the JavaScript, and then renders the content dynamically. Implementing SSR in AngularJS applications can lead to improved initial page load times and enhance the application's visibility to search engines. Let's delve into the concept of SSR, its benefits, and how it can be applied in AngularJS environments.
Benefits of Server-Side Rendering
Improved SEO
Search engines traditionally have a harder time indexing content that is rendered client-side since they need to execute JavaScript to see the content. SSR sends a fully rendered page to the client, ensuring that search engine crawlers can easily access and index the application's content, improving visibility and rankings.
Faster Initial Page Load
SSR speeds up the initial page load by sending a fully rendered page to the client. This reduces the time to first byte (TTFB) and the time it takes for users to see the first meaningful paint of the page, enhancing user experience, especially on slow connections or devices.
Enhanced Social Sharing
When sharing links on social media, SSR ensures that metadata (like images, titles, and descriptions) is correctly displayed, as the content is already rendered in the HTML. This can increase engagement and click-through rates from social platforms.
SSR Approaches in AngularJS
Angular Universal
Angular Universal is a technology that allows Angular apps to be rendered on the server. Although it's primarily associated with Angular (versions 2 and up), the concepts and underlying principles can be adapted for AngularJS applications with some effort. It pre-renders pages on the server, sending fully composed HTML to the client, which can significantly improve SEO and performance.
Ahead-of-Time (AOT) Compilation
Ahead-of-time (AOT) compilation converts AngularJS HTML and TypeScript code into efficient JavaScript code during the build phase before the browser downloads and runs the code. While AOT is more about optimizing the client-side execution, when combined with server-side rendering techniques, it can further reduce the initial load time by decreasing the JavaScript parsing and compilation time in the browser.
Hybrid Approaches
For AngularJS applications, implementing SSR might involve hybrid solutions or custom setups, leveraging tools like PhantomJS or Puppeteer to pre-render pages server-side. These tools can simulate a browser environment, execute JavaScript, and render the application as static HTML, which can then be served to the client. While not as streamlined as Angular Universal, these solutions offer a viable path for AngularJS applications to benefit from SSR.
Embracing Microservices Architecture in AngularJS Applications for Enhanced Scalability
Microservices architecture represents a paradigm shift in how applications are structured, promoting a more decentralized approach to application development. Unlike monolithic architectures, where all components of the application are tightly integrated and deployed as a single entity, microservices architecture breaks down the application into smaller, independently deployable services. Each service runs its own process and communicates with other services through well-defined APIs. This architectural style has gained popularity for its numerous advantages in scalability, maintainability, and flexibility, especially for complex applications. Let's explore how AngularJS applications can benefit from adopting a microservices architecture.
Advantages of Microservices Architecture
Enhanced Scalability
By decomposing an application into smaller, independent services, microservices architecture allows for targeted scaling of high-demand features without the need to scale the entire application. This can lead to more efficient use of resources and improved handling of user requests.
Improved Maintainability
Microservices promote modularity, making it easier to understand, develop, and test individual parts of the application. This modularity also simplifies updating and adding new features, as changes to one service do not necessarily impact others.
Flexibility in Technology Stack
Microservices architecture allows different services to be written in different programming languages, use different data storage technologies, and adopt new technologies without affecting the entire system. This flexibility can be particularly advantageous for AngularJS applications looking to integrate with services in languages that offer better performance for specific tasks.
Integrating Microservices with AngularJS Applications
Breaking Down the Application
The first step in adopting a microservices architecture is to identify logical modules within your AngularJS application that can function as independent services. Common separation criteria include business functionality (e.g., user management, payment processing), data entity management, or specific functionality that requires scalability.
Managing Communication Between Services
Services in a microservices architecture communicate with each other using lightweight protocols, typically over HTTP/REST or message queues for asynchronous communication. For AngularJS applications:
- Frontend to Microservice Communication: The AngularJS frontend can interact with microservices through RESTful APIs. AngularJS's $http service or the more advanced $resource service can be used to make these HTTP requests.
- Service-to-Service Communication: For backend microservices, adopting an API Gateway pattern can simplify the communication from the AngularJS frontend by routing requests to the appropriate microservice. This can also abstract the complexity of direct service-to-service communication from the front end.
Mastering Scalability in AngularJS Applications: Best Practices and Strategic Insights
Crafting AngularJS applications that are primed for scalability and efficiency requires adherence to a set of best practices, from the organization of code to the optimization of databases. As applications grow in complexity and user base, these practices become indispensable in ensuring performance, maintainability, and a seamless user experience. Let's delve into these best practices and consider additional strategies crucial for large-scale deployments.
Best Practices for Scalable AngularJS Applications
Code Organization and Modularity
- Follow the Component-Based Architecture: Organize your application into reusable components. This approach not only makes the application easier to maintain but also facilitates lazy loading, which can significantly improve load times.
- Leverage AngularJS Modules: Use modules to encapsulate and manage different parts of your application, such as services, controllers, and directives. This enhances code readability and makes it easier to manage dependencies.
Efficient Dependency Management
- Minimize Global Dependencies: Use dependency injection, a core feature of AngularJS, to manage your application's components. This reduces global dependencies and makes it easier to test and reuse components.
- Keep Third-Party Libraries to a Minimum: While third-party libraries can add functionality quickly, they also increase the application's size and can introduce security vulnerabilities. Evaluate the necessity of each library and consider lightweight alternatives.
Additional Considerations for Large-Scale Deployments
Load Balancing
Implementing load balancing distributes incoming traffic across multiple servers, preventing any single server from becoming a bottleneck. This is crucial for maintaining high availability and handling peak loads efficiently.
Database Optimization
- Indexing: Proper indexing can dramatically improve query performance by reducing the data scanned.
- Normalization and Denormalization: Balance normalization and denormalization to optimize read and write operations. Denormalization can speed up read operations, which is often critical for user-facing applications.
- Caching: Use caching strategies to store frequently accessed data in memory, reducing the number of direct database queries and thereby decreasing response times.
Conclusion
Building scalable and high-performance web applications with AngularJS is an art that combines strategic planning, efficient code organization, and the adoption of modern architectural practices. Throughout this article, we've explored the foundations of scalable AngularJS applications, from understanding the core components and optimizing performance to embracing server-side rendering and microservices architecture. We delved into best practices for code organization, dependency management, testing strategies, and additional considerations crucial for managing large-scale deployments effectively.
Collaborate with expert
Angular JS Development Service Agencies for dynamic web solutions.
share this page if you liked it 😊
Other Related Blogs
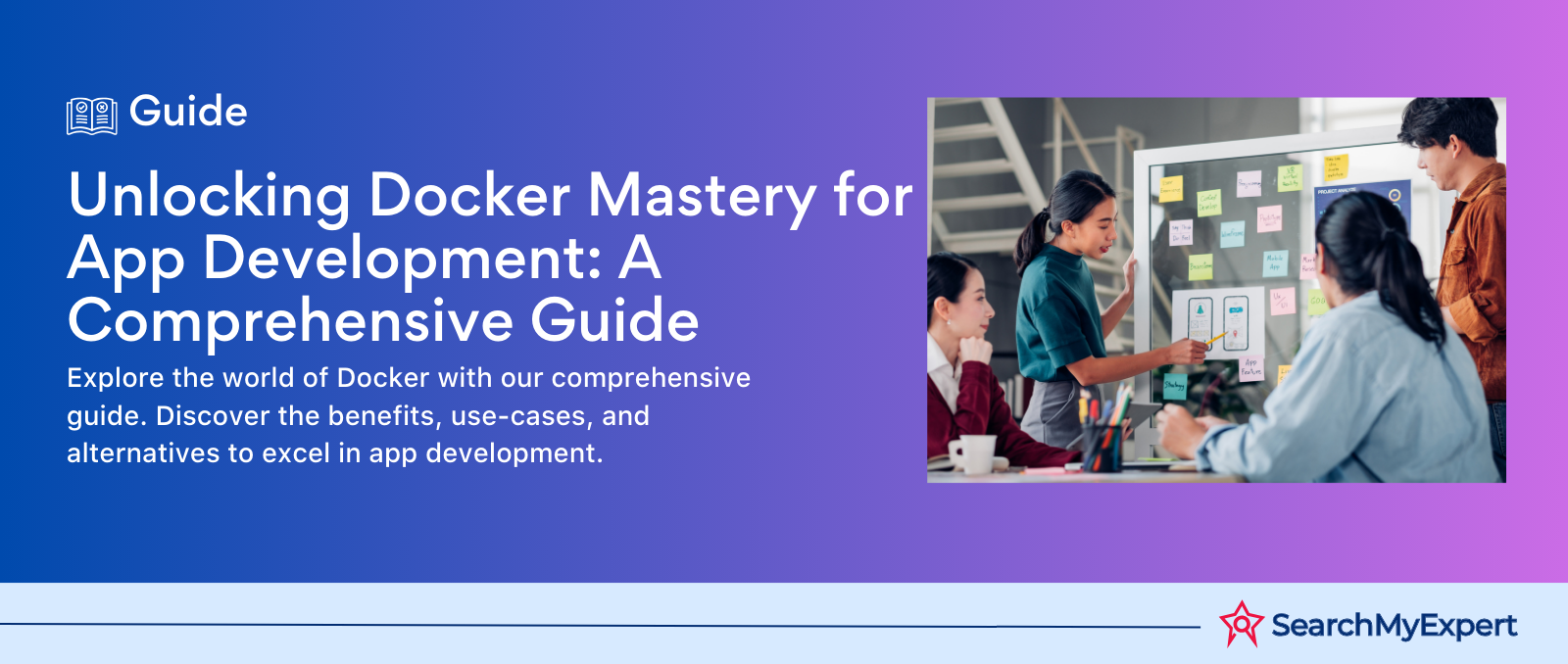
Mastering Docker for App Development: A Comprehensive Guide to Benefits, Use-Cases, and Alternatives
STAY UP TO DATE
GET PATH'S LATEST
Receive bi-weekly updates from the SME, and get a heads up on upcoming events.
Contact Us
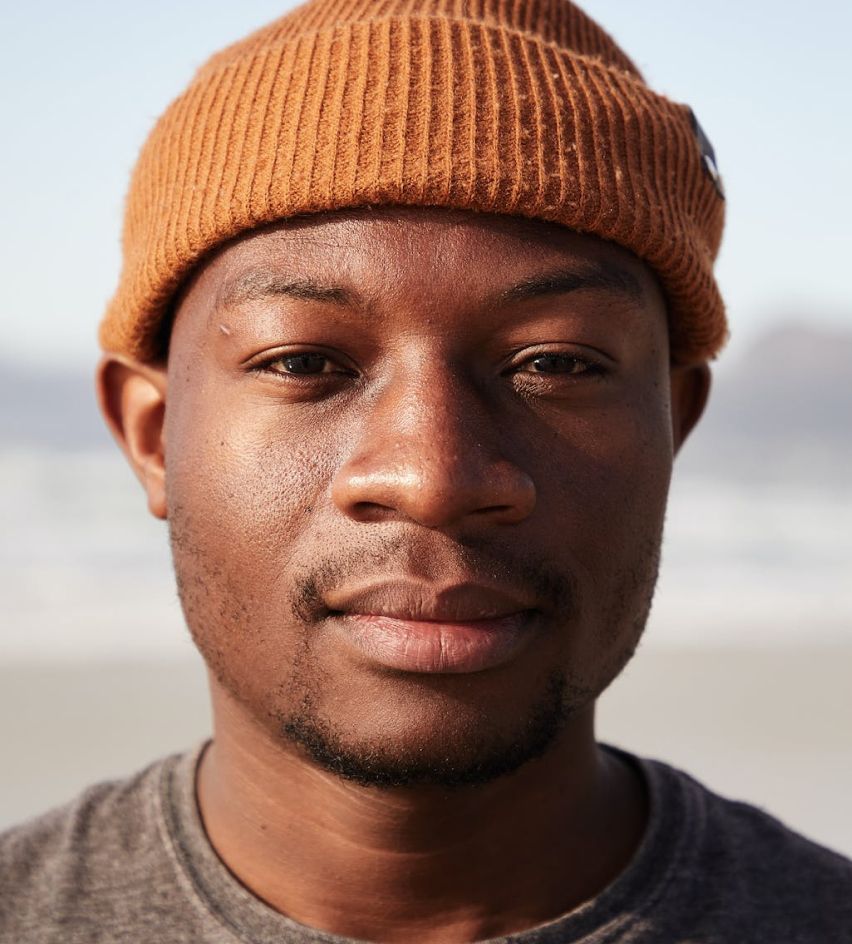
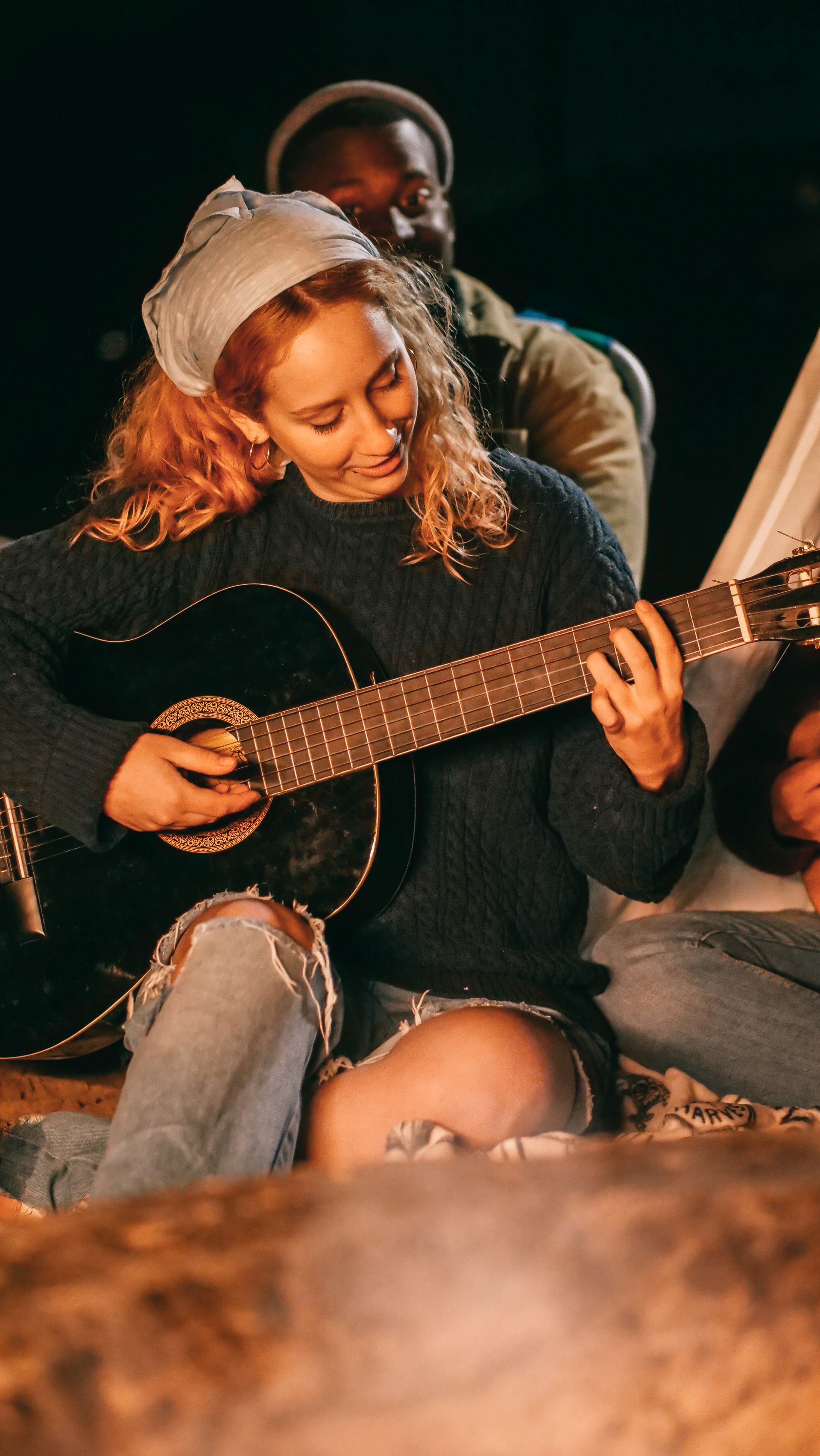