Further PHP Object-Oriented Programming: Methods, Structures, and Upcoming Developments
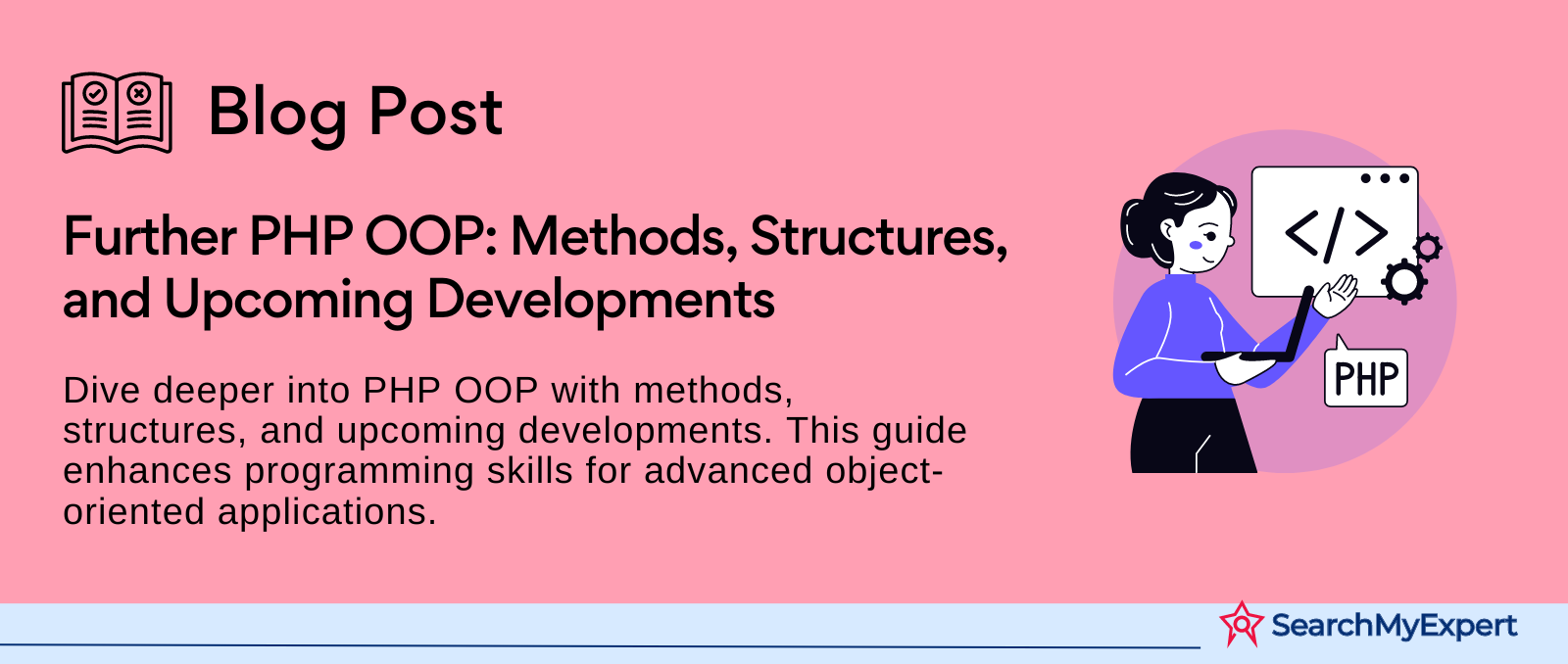
Introduction to Advanced Object-Oriented Programming Concepts
Object-oriented programming (OOP) stands as a foundational concept in modern software development, offering a structured approach to coding that is both efficient and scalable. As we delve into the world of Advanced OOP, it's crucial to build upon the core principles of OOP: classes, objects, methods, and properties. These elements form the backbone of basic object-oriented design, providing a framework that supports the development of robust and maintainable software.
Recap of Core OOP Principles
- Classes: At the heart of OOP lie classes. Think of a class as a blueprint for creating objects. It encapsulates data for the object and methods to manipulate that data. For instance, in a banking application, a BankAccount class might include data such as balance and methods like deposit() and withdraw().
- Objects: Objects are instances of classes. Continuing with our example, if BankAccount is a class, then each individual account - John's account, Mary's account - are object of the BankAccount class, each with their own distinct data.
- Methods: Methods are functions defined within a class that describe the behaviors of an object. In our BankAccount class, the deposit() method might increase the account balance by the amount deposited.
- Properties: Properties are variables contained in a class that hold the data of an object. In the BankAccount example, balance is a property that stores the monetary amount in the account.
Transitioning from Basic to Advanced Object-Oriented Design
As developers gain proficiency in these basic principles, the transition to advanced OOP becomes essential, especially when dealing with complex, large-scale projects. Advanced OOP involves more sophisticated techniques such as inheritance, polymorphism, abstraction, and encapsulation. These techniques not only enhance code readability and reuse but also significantly improve the maintainability of the software.
Benefits of Utilizing Advanced OOP Techniques for Complex Projects
- Improved Code Reusability and Efficiency: Through inheritance and polymorphism, developers can create a general class and extend or modify it for specific needs, avoiding code duplication.
- Enhanced Code Maintainability: Abstraction and encapsulation hide the complex internal workings of a class, exposing only what is necessary. This separation of concerns makes it easier to manage and update the software.
- Scalability and Flexibility: Advanced OOP makes it easier to scale and adapt software as requirements evolve. It allows for the development of modules that can be easily modified or replaced without affecting the entire system.
- Better Collaboration Among Developers: With a well-structured OOP approach, different teams can work on different modules or classes independently, streamlining the development process.
- Easier Debugging and Testing: Since advanced OOP promotes modular code, it becomes simpler to test and debug individual components without impacting the whole application.
Deep Dive into Inheritance and Polymorphism
Inheritance and polymorphism stand as the cornerstones of advanced Object-Oriented Programming (OOP). These concepts not only streamline code but also enhance its flexibility and maintainability. In this section, we will explore the intricacies of advanced inheritance techniques, including multiple inheritance, abstract classes, and interfaces, and delve into the practical applications and benefits of polymorphism.
Advanced Inheritance Techniques
Multiple Inheritance:
- Definition: Multiple inheritance allows a class to inherit features from more than one parent class. This technique can lead to a more flexible and comprehensive class structure.
- Example: Consider a scenario where a FlyingCar class inherits properties from both Car and Aircraft classes, combining functionalities like drive() from Car and fly() from Aircraft.
Abstract Classes:
- Definition: An abstract class is a class that cannot be instantiated on its own and is typically used to define a common interface for its subclasses.
- Example: An Animal abstract class may have an abstract method makeSound(). Subclasses like Dog and Cat would implement this method with their specific sound behaviors.
Interfaces:
- Definition: An interface is a completely abstract class that defines a set of methods that the implementing class must provide.
- Example: An IChargeable interface might declare a method chargeBattery(). Any class like ElectricCar that implements this interface would need to provide the actual implementation of chargeBattery().
Utilizing Inheritance to Extend Functionality and Build Reusable Code
- Code Reusability: Inheritance promotes code reusability. For example, a BaseVehicle class can define common functionalities shared by all vehicles, such as startEngine() or stopEngine(), which can then be inherited by subclasses like Truck, Bike, etc.
- Extending Functionality: Subclasses can not only inherit properties and methods from their parent classes but can also add new features or override existing ones. For instance, a SportsCar class inheriting from Car might include additional methods like activateTurbo().
Understanding Polymorphism and Its Benefits
What is Polymorphism?:
- Polymorphism in OOP allows objects of different classes to be treated as objects of a common superclass. It is primarily of two types: compile-time (method overloading) and runtime (method overriding).
Dynamic Method Dispatch:
- This is a mechanism by which a call to an overridden method is resolved at runtime rather than compile-time. It allows for more flexible and dynamic code.
- For example, consider a function that takes a Shape object and calls its draw() method. Whether the shape is a circle, square, or triangle, the correct draw() method is called, depending on the actual object type.
Flexibility and Maintainability:
- Polymorphism enhances flexibility and maintainability. It allows developers to write more generic and abstract code, making it easier to extend and maintain.
- For instance, a single method can be written to handle an array of Shapes, and regardless of the specific shape type, the method will correctly process each shape, adhering to the specific behavior of its class.
Mastering Class Design Patterns
Design patterns are standardized solutions to common problems in software design. They provide a blueprint for how to structure and solve various issues, thereby enhancing code readability, reusability, and maintainability. In this section, we'll explore some popular class design patterns — Factory, Singleton, Adapter, and Observer — and discuss their application in solving specific coding challenges, particularly focusing on best practices for their implementation in PHP.
Introduction to Popular Design Patterns
Factory Pattern:
- Concept: The Factory pattern is used to create objects without specifying the exact class of object that will be created.
- Use-case: This pattern is particularly useful when the exact types of objects need to vary based on input or application configuration.
Singleton Pattern:
- Concept: The Singleton pattern ensures that a class has only one instance and provides a global point of access to it.
- Use-case: It's widely used for managing database connections or application configurations where a single shared resource is preferable.
Adapter Pattern:
- Concept: The Adapter pattern allows incompatible interfaces to work together. It acts as a bridge between two incompatible interfaces.
- Use-case: This pattern is useful when integrating new components into an existing system that expects different interfaces.
Observer Pattern:
- Concept: The Observer pattern defines a dependency between objects so that when one object changes its state, all its dependents are notified.
- Use-case: It's commonly used in implementing event handling systems.
Applying Design Patterns to Solve Coding Challenges
Factory Pattern:
- Application: In a PHP web application, a Factory pattern can be used to create different types of response objects based on the request type (HTML, JSON, XML).
- Advantage: Simplifies code by abstracting the object creation process.
Singleton Pattern:
- Application: Implement a Singleton for database connections in PHP to ensure that only one connection is active at any given time.
- Advantage: Reduces overhead and ensures consistent access to the resource.
Adapter Pattern:
- Application: Integrate a third-party library with a different interface into an existing PHP application.
- Advantage: Increases compatibility without altering existing codebase.
Observer Pattern:
- Application: Implement event listeners in a PHP application, where a change in the user model (e.g., updating user data) triggers various follow-up actions.
- Advantage: Promotes loose coupling and enhances flexibility.
Best Practices for Implementing Design Patterns in PHP
- Understand the Problem Clearly: Before choosing a design pattern, thoroughly understand the problem and the pattern’s applicability.
- Use Patterns Appropriately: Do not force the use of a pattern if it does not fit naturally within the application’s architecture.
- Keep It Simple: Sometimes, the simplest solution is the best. Overusing design patterns can lead to unnecessary complexity.
- Stay Consistent: Once a pattern is chosen, apply it consistently across the application to maintain code readability and integrity.
- Documentation: Document the use of design patterns in the code for future reference and maintenance.
- Refactor as Needed: Be open to refactoring if a pattern implementation no longer suits evolving application needs.
Exploring Error Handling and Exception Management
Error handling and exception management are critical components of robust software development. Properly managing errors not only ensures greater fault tolerance but also enhances the reliability and maintainability of the application. In this section, we will explore the concepts of throwing and catching exceptions, customizing exception handling, and leveraging error logging and reporting mechanisms, with a focus on their practical implementation for effective debugging.
Throwing and Catching Exceptions
Basics of Exception Handling:
- Concept: Exception handling in programming involves capturing errors that occur during the execution of a program and responding to them without stopping the entire process.
- Implementation: In many programming languages, this is achieved using try-catch blocks where the code that might generate an error is placed inside a try block, and the handling of any exceptions is done in the catch block.
Advantages:
- Robust Error Handling: By catching exceptions, programs can gracefully handle errors, ensuring that the application remains stable and operational.
- Fault Tolerance: It helps in creating fault-tolerant systems that can manage unexpected situations without crashing.
Customizing Exception Handling
User-Defined Exceptions:
- Concept: Developers can create custom exception classes that extend the base Exception class. This allows for more specific error handling.
- Application: For example, a DatabaseConnectionException class can be created to handle errors specifically related to database connections.
Exception Classes:
- Details: Exception classes can contain additional information like error codes, messages, or methods to retrieve more details about the exception.
- Benefit: This customization provides more control over error management and allows for more detailed error reporting.
Leveraging Error Logging and Reporting Mechanisms
Error Logging:
- Purpose: Logging errors is crucial for diagnosing and fixing issues in software. It involves recording errors and exceptions into a file or a logging system.
- Tools: Tools like Log4j for Java or Monolog for PHP can be utilized for effective error logging.
Error Reporting:
- Functionality: Error reporting involves the generation of reports based on logged errors, which can then be used for debugging and improving the software.
- Strategies: Implementing strategies like email notifications for critical errors or dashboard displays for error metrics can be highly effective.
Best Practices:
- Comprehensive Logging: Ensure that error logs are detailed, including timestamps, error messages, and context.
- Monitoring and Alerts: Set up monitoring systems that alert developers to critical issues in real time.
- Regular Analysis: Regularly analyze error logs to identify patterns and areas for improvement.
Implementing Design Principles for Maintainable Code
Maintainable code is the cornerstone of successful software development, enabling efficient future development and collaboration. One of the key methodologies to achieve this is through the implementation of SOLID principles in Object-Oriented Programming (OOP). These principles guide developers in writing clean, efficient, and modular code. In this section, we will explore each of these principles and how they contribute to improving code readability and maintainability.
SOLID Principles
Single Responsibility Principle (SRP):
- Concept: A class should have only one reason to change, meaning it should only have one job or responsibility.
- Application: For instance, in a user management system, separate classes should be responsible for user data handling and user authentication.
Open/Closed Principle (OCP):
- Concept: Software entities (classes, modules, functions, etc.) should be open for extension, but closed for modification.
- Application: Implementing this can be done by using abstract classes or interfaces, allowing new functionalities to be added without altering existing code.
Liskov Substitution Principle (LSP):
- Concept: Objects of a superclass should be replaceable with objects of its subclasses without affecting the correctness of the program.
- Application: Ensure subclass methods fully match the behavior and expectations set by the superclass.
Interface Segregation Principle (ISP):
- Concept: No client should be forced to depend on methods it does not use.
- Application: Instead of one large interface, multiple specific interfaces are better suited for clients that require only a portion of the interface’s functionality.
Dependency Inversion Principle (DIP):
- Concept: High-level modules should not depend on low-level modules. Both should depend on abstractions.
- Application: For example, a service class should depend on an abstract layer rather than a concrete database access class.
Writing Clean, Efficient, and Modular Code
- Adherence to OOP Best Practices: Following OOP best practices like encapsulation, abstraction, and polymorphism, in conjunction with SOLID principles, leads to a well-structured and organized codebase.
- Code Modularity: Breaking down the code into smaller, manageable, and interchangeable modules or components makes the system more flexible and easier to understand.
- Efficiency: Efficient code is not just about performance but also about simplicity and clarity. Avoiding over-complication and sticking to the problem at hand is key.
Improving Code Readability and Maintainability
Consistent Coding Standards:
- Adopting and sticking to coding standards and conventions is essential for ensuring that code is understandable and maintainable by all team members.
Documentation and Comments:
- Proper documentation and meaningful comments help in understanding the purpose and functionality of the code, making maintenance easier.
Regular Refactoring:
- Periodically revisiting and refactoring code to improve its structure and efficiency without changing its external behavior is crucial for long-term maintainability.
Peer Reviews and Collaborative Coding:
- Regular peer reviews and collaborative coding practices ensure collective code ownership and help in catching potential issues early
Optimizing Object Performance and Memory Management
In the realm of software development, particularly in languages like PHP, optimizing object performance and memory management is crucial for building efficient and scalable applications. Understanding how memory allocation works and how to effectively manage memory can significantly enhance the performance of your applications. This section focuses on key aspects of memory allocation and garbage collection in PHP, techniques for memory optimization, and balancing these considerations with object-oriented design principles.
Understanding Memory Allocation and Garbage Collection in PHP
Memory Allocation in PHP:
- Overview: PHP manages memory through an internal mechanism that allocates memory to objects and variables as needed.
- Process: When an object is created in PHP, memory is allocated to store it. As more objects and variables are created, more memory is consumed.
Garbage Collection:
- Function: Garbage collection is the process of identifying and freeing up memory that is no longer in use.
- Mechanism: PHP's garbage collector periodically checks for objects that are no longer referenced and releases their memory.
Techniques for Memory Optimization
Reference Counting:
- Concept: PHP uses reference counting to keep track of how many references exist to a particular object.
- Strategy: Proper management of references, like unsetting variables or using references judiciously, can help in optimizing memory usage.
Garbage Collection Tuning:
- Approach: Tuning the garbage collector involves adjusting certain parameters, like the frequency of garbage collection cycles, to better suit the application's needs.
- Best Practice: Monitor the application's performance to determine the optimal garbage collection settings.
Memory Leaks Detection:
- Tools: Use tools like Xdebug or Zend Debugger to detect memory leaks in PHP applications.
- Practice: Regularly check for memory leaks, especially in long-running scripts or applications with complex data structures.
Balancing Performance Considerations with Object-Oriented Design Principles
Efficient Object Creation:
- Consideration: While creating objects is central to OOP, unnecessary object creation can lead to memory bloat.
- Balance: Use design patterns like Flyweight for managing shared objects efficiently.
Lazy Loading:
- Technique: Lazy loading defers the initialization of an object until it’s needed.
- Impact: This can improve performance by reducing memory usage and speeding up application start times.
Resource Management:
- Aspect: Efficiently managing resources like database connections and file handles is crucial.
- Method: Implementing resource management patterns and ensuring proper closure of resources can minimize memory leaks.
Profiling and Optimization:
- Action: Regularly profile the application for performance bottlenecks.
- Methodology: Use profiling tools to identify memory-intensive sections and optimize them without compromising OOP principles.
Advanced Topics and Future of OOP in PHP
Object-oriented programming (OOP) in PHP continues to evolve, embracing advanced features that streamline code organization, promote reuse, and align with modern programming practices. In this section, we delve into two significant advancements: namespaces and traits, explore PHP frameworks that exemplify advanced OOP concepts, and discuss the latest developments and trends within the PHP OOP ecosystem.
Exploring Namespaces and Traits
Namespaces:
- Purpose: Namespaces in PHP are designed to solve two primary problems: name collisions between code you create, and internal PHP classes/functions/constants, and to provide a way to group related classes, interfaces, functions, and constants.
- Usage: Namespaces are declared using the namespace keyword, allowing for better organization of large applications and libraries, and avoiding name conflicts in large projects.
Traits:
- Concept: Traits are a mechanism for code reuse in single inheritance languages like PHP. They offer a set of methods that you can use in multiple classes.
- Application: Traits are similar to classes, but are intended to group functionality in a fine-grained and consistent way. They can be included within classes using the use keyword.
Introduction to PHP Frameworks Based on Advanced OOP Concepts
Laravel:
- Overview: Laravel is a modern PHP framework that follows the MVC (Model-View-Controller) pattern and utilizes advanced OOP concepts. It offers elegant syntax and helps in creating scalable and maintainable applications.
- Features: Laravel includes features like Eloquent ORM for database operations, a Blade templating engine, and extensive libraries for tasks like routing, sessions, and caching.
Symfony:
- Details: Symfony is another PHP framework that leverages OOP principles. Known for its flexibility, Symfony allows developers to pick the specific components they need for a project.
- Components: Symfony is famous for its reusable components, which can be integrated into any PHP application.
Latest Developments and Trends in Object-Oriented Programming Within the PHP Ecosystem
PHP 8 Features:
- New Additions: The release of PHP 8 has introduced features like Union Types, Named Arguments, Match Expressions, and Attributes (Annotations), enhancing the OOP capabilities of PHP.
- Performance Improvements: JIT (Just In Time) compilation in PHP 8 has significantly improved performance, making PHP a more robust choice for complex OOP-based applications.
Increased Focus on Type Safety:
- Trend: There's a growing trend in the PHP community to improve type safety with features like strict typing and type declarations, which lead to more robust and maintainable code.
Emphasis on PSR Standards:
- Standardization: PHP-FIG (PHP Framework Interop Group) provides PSR (PHP Standard Recommendations) standards, promoting consistency and interoperability across different PHP frameworks and projects.
Integration with Emerging Technologies:
- Future Direction: PHP continues to evolve with integration into modern technologies like cloud services, machine learning, and IoT, expanding its OOP capabilities to new domains.
Conclusion
The journey through advanced Object-Oriented Programming in PHP has revealed a landscape rich with possibilities and innovations. From the strategic use of namespaces and traits to enhance code organization and reuse, to the exploration of powerful frameworks like Laravel and Symfony, PHP's OOP capabilities are more robust than ever. The latest developments in the language, particularly with the advent of PHP 8, are pushing the boundaries of performance, type safety, and interoperability.
As PHP continues to integrate with cutting-edge technologies and adhere to evolving standards, it stands poised to maintain its relevance and efficiency in the face of modern software development challenges. For developers, staying attuned to these advancements is not just beneficial; it's essential for crafting state-of-the-art, scalable, and maintainable applications. The future of OOP in PHP is bright, and it beckons with opportunities for innovation, efficiency, and sophisticated software solutions.
Navigate the future of web development with
PHP Development Service Agencies.
share this page if you liked it 😊
Other Related Blogs
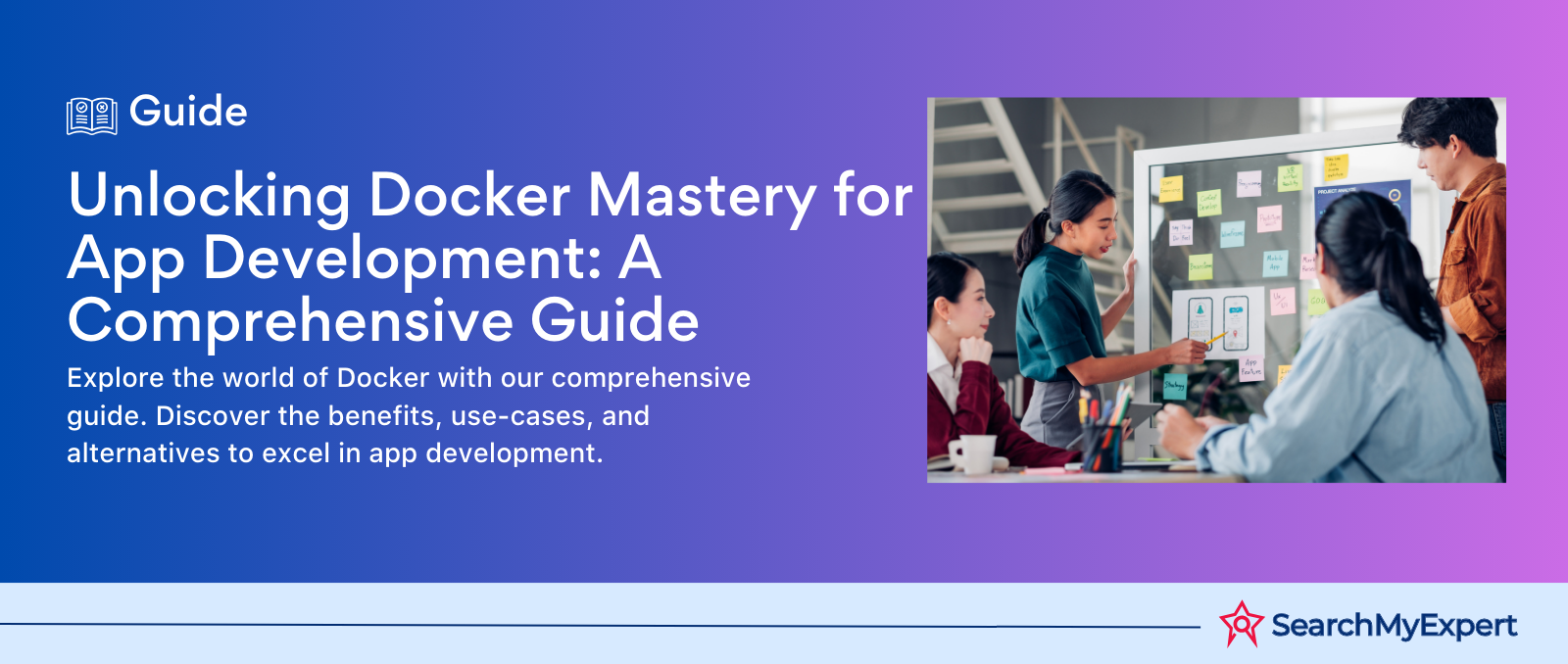
Mastering Docker for App Development: A Comprehensive Guide to Benefits, Use-Cases, and Alternatives
STAY UP TO DATE
GET PATH'S LATEST
Receive bi-weekly updates from the SME, and get a heads up on upcoming events.
Contact Us
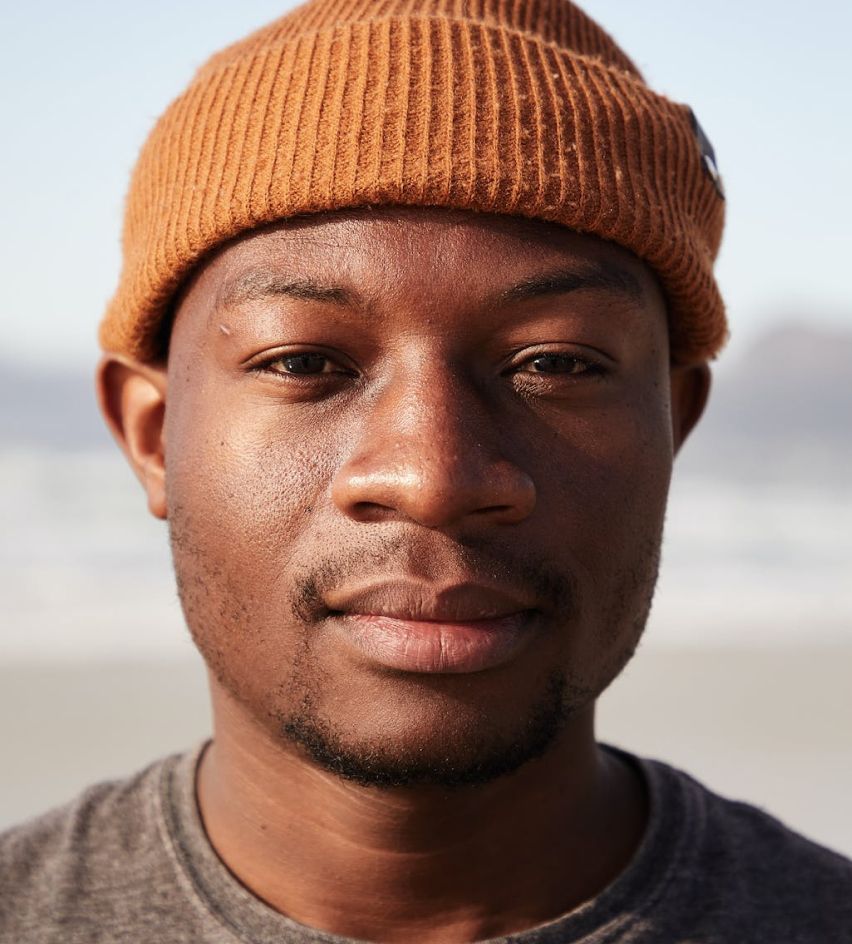
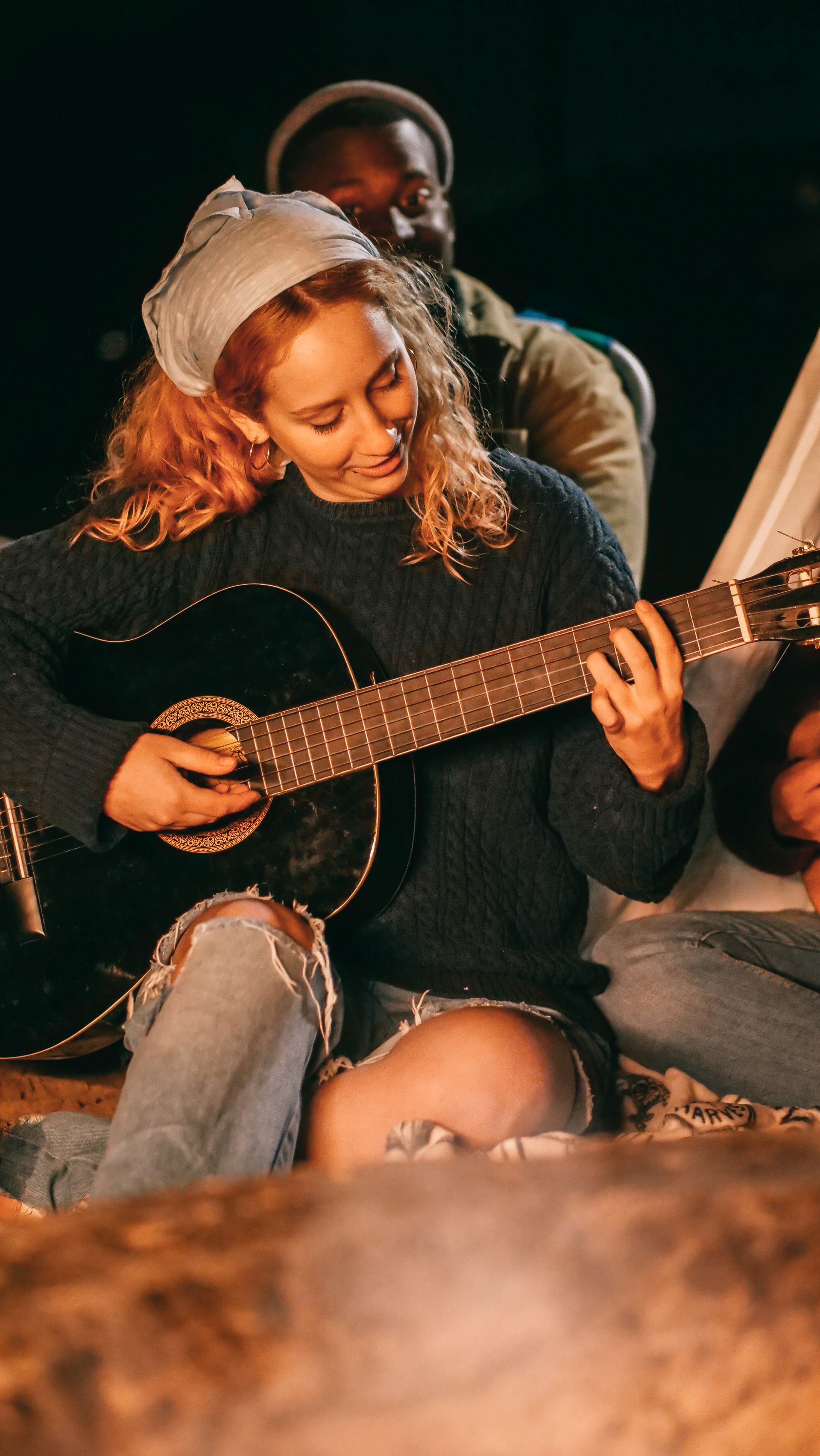