Node.js Module Optimisation: Development & Maintaining Advice
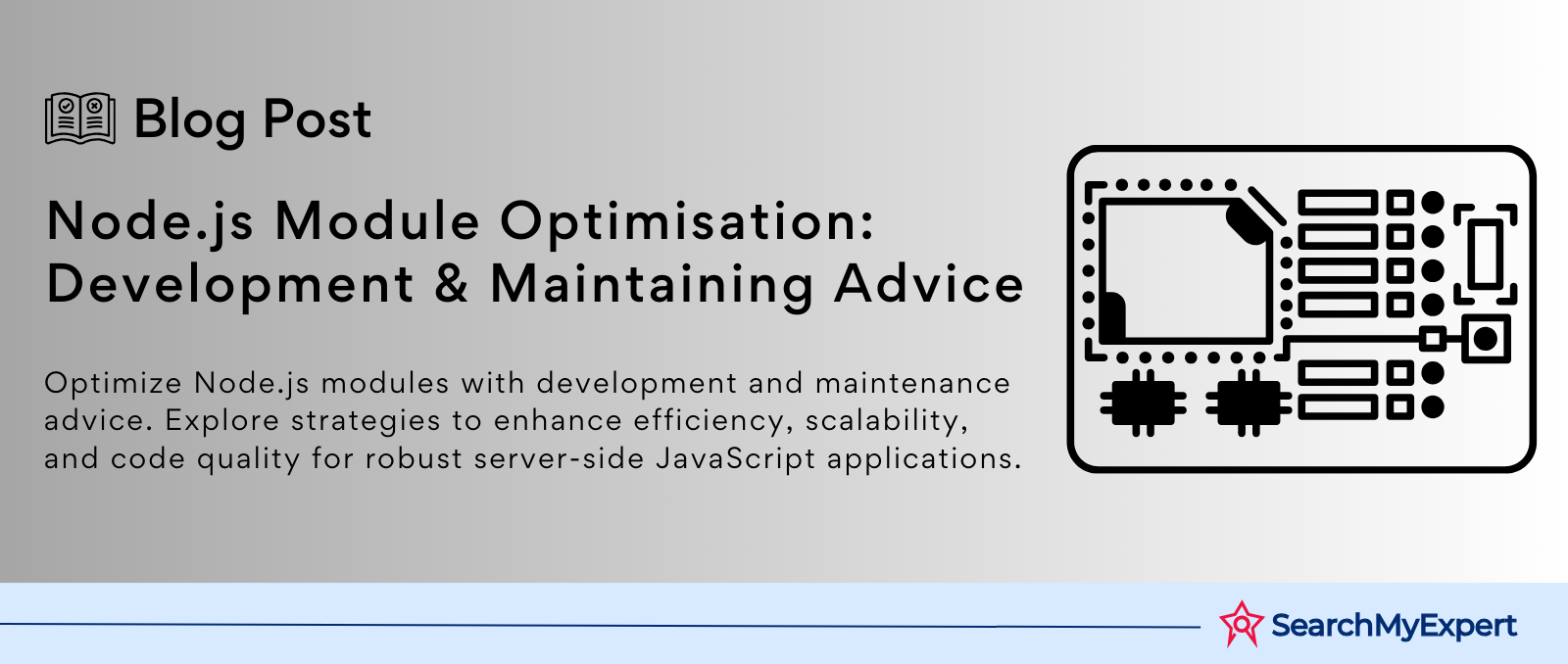
Understanding Node.js Modules
Node.js Modules
Node.js has revolutionized the way we develop applications by enabling JavaScript to run on the server side. At the heart of this powerful platform lies a simple yet profoundly flexible concept: modules. In this section, we delve into what modules are, their significance in Node.js development, and the key benefits they bring to the table, including code organization, reusability, and maintainability. Additionally, we explore the different types of modules available to Node.js developers: built-in (core) modules and third-party (npm packages).
What are Modules?
In Node.js, modules are discrete units of code that can be separated from the main application to enhance functionality without cluttering the global namespace. Think of modules as individual pieces of a puzzle. Each piece (module) has a specific role and can be developed, maintained, and updated independently. This modularity allows developers to build highly scalable and manageable applications.
Significance of Modules in Node.js Development
Modules are not just a convenience; they are a fundamental aspect of Node.js architecture. They encourage a clean separation of concerns, making applications easier to understand, debug, and extend. By using modules, developers can isolate specific functionalities, making the codebase more organized and efficient.
Key Benefits of Using Modules
- Code Organization: Modules help in structuring the code more effectively. By dividing the application into smaller, manageable pieces, developers can easily navigate and manage the codebase.
- Reusability: Once a module is created, it can be reused across different parts of an application or even in different projects, saving time and effort in the development process.
- Maintainability: Modules make it easier to update and maintain applications. Changes to a module do not affect the entire application, reducing the risk of breaking other parts of the code.
Different Types of Modules
- Built-in (Core) Modules: Node.js comes with a set of core modules that are part of the platform itself. These modules provide basic functionalities required by most applications, such as file system operations, HTTP networking, and more.
- Third-party (npm packages): The Node.js community has contributed a vast ecosystem of third-party modules, available through the npm (Node Package Manager) registry. These packages can add powerful features to your applications, from web frameworks like Express to utilities for data manipulation.
Creating Your Own Modules
When developing with Node.js, creating your own modules is a fundamental practice that enables you to structure your application efficiently. This section will guide you through the essentials of constructing a Node.js module, including its structure, the definition of its components, and the methods for exporting these components for use in other parts of your application. Additionally, we will cover best practices for ensuring your modular code is clear, cohesive, and maintainable.
Structure of a Node.js Module
A Node.js module is essentially a JavaScript file that encapsulates specific functionality or a set of functionalities. The power of a module comes from its ability to export this functionality, making it accessible to other parts of your application. Each module has its own context, meaning that variables or functions defined in a module won't pollute the global scope.
Defining Functions, Variables, and Classes Within Modules
Within your module, you can define any number of functions, variables, or classes. These definitions form the core of your module, providing the specific functionalities that you wish to encapsulate. The beauty of modules lies in their encapsulation; the inner workings of your module remain hidden from the outside world, exposing only what you choose to share.
Exporting Elements for Use in Other Modules
To make the functionalities of your module available to other parts of your application, you need to export them. Node.js provides two main ways to accomplish this:
- module.exports: This method is used when you want to export a single object, class, or function from your module.
- exports: This is a reference to module.exports and can be used to export individual functionalities by attaching them as properties of exports.
The choice between module.exports and exports depends on how you want to structure your module's output. Whether you're exporting a single class or multiple functions and variables, Node.js offers the flexibility to structure your module exports according to your project's needs.
Best Practices for Writing Modular Code
Adhering to certain best practices can significantly improve the clarity, cohesion, and maintainability of your modular code:
- Focus on Cohesion: Each module should have a single, well-defined purpose. Group related functionalities together to maintain cohesion within your modules.
- Encapsulation: Leverage encapsulation by exposing only necessary parts of your module. This approach keeps the module's interface clean and straightforward.
- Descriptive Naming: Choose clear and descriptive names for your modules, functions, and variables. Good naming conventions enhance readability and make your code more understandable.
- Document Your Code: Effective documentation is crucial, especially for modules that are intended for use by others. Clearly document the purpose of the module and how to use its exported functionalities.
- Modularize Thoughtfully: While modules are powerful, over-modularization can lead to fragmentation and complexity. Strike a balance by modularizing your codebase in a way that improves its structure without introducing unnecessary overhead.
Importing and Using Modules
The ability to import and utilize modules is a cornerstone of Node.js development, enabling developers to incorporate a wide range of functionalities into their applications with ease. This process is primarily facilitated through the require() function, which allows for the integration of both custom and third-party modules. Understanding how to effectively import and use these modules, as well as navigate potential pitfalls such as circular dependencies, is crucial for building robust Node.js applications.
Using the require() Function to Import Modules
The require() function is the standard approach for importing modules in Node.js. It allows developers to include the contents of one file in another, enabling the reuse of code across the application. When invoking require(), the path to the module file or the name of the module is specified, and the module's exported contents are made available for use. This mechanism is used for importing a wide range of modules, from those you have created yourself to built-in Node.js modules and third-party libraries available through npm.
Accessing Exported Elements Using Dot Notation
Once a module is imported using require(), its exported elements, such as functions, objects, or classes, can be accessed using dot notation. This direct access facilitates a modular approach to application development, where functionalities are compartmentalized and can be easily incorporated and reused throughout the application. By adhering to this structured approach, developers can enhance code readability and maintainability, promoting a clean and efficient development process.
Understanding Potential Issues with Circular Dependencies
Circular dependencies represent a complex challenge in module-based development, occurring when two or more modules depend on each other to function. This interdependency can lead to issues where modules are not fully loaded before they are used, potentially resulting in runtime errors or undefined behaviors.
To mitigate the risks associated with circular dependencies, developers should aim for a modular design that minimizes direct interdependencies between modules. This can involve reevaluating the application's architecture to ensure a more linear and hierarchical module structure. Additionally, developers can employ strategies such as lazy loading or dependency injection to manage dependencies more effectively, thereby reducing the likelihood of encountering circular dependency issues.
Working with the npm Package Manager
The npm (Node Package Manager) plays a pivotal role in the Node.js ecosystem, serving as the central repository for hosting and sharing Node.js packages. It not only facilitates the discovery and installation of third-party modules but also assists in managing project dependencies efficiently. In this section, we will explore the functionalities of npm, including how to search for and install third-party modules, manage dependencies through package.json and package-lock.json, and update or uninstall modules using npm commands.
Introduction to npm and its Role in Node.js Module Ecosystem
npm is more than just a package manager; it's a comprehensive platform for publishing, discovering, and installing Node.js packages. It significantly simplifies the process of sharing code across projects and teams, making it easier to extend the functionality of Node.js applications by incorporating a wide array of libraries and tools available in the npm registry.
Searching for and Installing Third-Party Modules from the npm Registry
The npm registry (https://www.npmjs.com/) hosts thousands of packages for various functionalities, from web frameworks to utility libraries. Developers can search for packages directly on the npm website or use the npm CLI (Command Line Interface) with commands like npm search to find packages relevant to their needs. Once a suitable package is found, it can be easily installed into a project using the npm install <package-name> command. This command not only downloads the package but also adds it as a dependency in the project's package.json file, ensuring that other developers working on the project can install the same dependencies with ease.
Managing Dependencies in Your Projects Using package.json and package-lock.json
The package.json file is a vital component of any Node.js project managed with npm. It keeps track of all the project's dependencies, specifying the versions of third-party modules the project relies on. When a package is installed, npm updates the package.json file accordingly, providing a clear overview of the project's dependencies.
The package-lock.json file, on the other hand, locks down the exact versions of all packages and their dependencies installed in the project. This file ensures that all team members and deployment environments use the same versions of each package, mitigating the risks associated with version discrepancies.
Updating and Uninstalling Modules with npm Commands
Maintaining the currency and health of a project's dependencies is crucial. npm provides commands such as npm update to update the packages to their latest versions based on the version ranges specified in package.json. When a package is no longer needed, it can be removed from the project using the npm uninstall <package-name> command, which also removes its entry from the package.json file, keeping the project clean and up-to-date.
Advanced Module Concepts
In the evolving landscape of Node.js development, understanding the intricacies of module systems is crucial for modern application architecture. This section delves into the two predominant module systems in JavaScript: CommonJS (CJS) and ES Modules (ESM). We will explore their differences, practical applications, and the concept of dynamic imports, along with asynchronous module loading techniques to enhance your Node.js projects.
Understanding CommonJS (CJS) and ES Modules (ESM) Module Systems
CommonJS (CJS): CommonJS is the standard module system in Node.js for encapsulating and organizing JavaScript code. It allows developers to export and require modules synchronously using the require() function and module.exports. CommonJS is widely used in server-side development and is known for its simplicity and ease of use in synchronous operations.
ES Modules (ESM): ES Modules is the official standard for JavaScript modules in the ECMAScript specification. It introduces an import and export syntax for loading modules asynchronously, offering a more flexible and forward-looking approach compared to CommonJS. ESM is designed to be compatible across different environments, including browsers and Node.js, making it suitable for universal JavaScript applications.
Differences Between Them and When to Use Which
The key differences between CommonJS and ES Modules lie in their syntax and loading mechanism:
- Syntax: CommonJS uses require() for importing modules and module.exports for exporting, whereas ES Modules use the import and export keywords.
- Module Loading: CommonJS modules are loaded synchronously, making them ideal for server-side applications where modules are stored locally. ES Modules, on the other hand, support asynchronous loading, which is advantageous for web applications that benefit from non-blocking operations.
The choice between CommonJS and ES Modules often depends on the application's context and requirements:
Use CommonJS when:
- Your project is Node.js-based and doesn't need to run in a browser environment.
- You prefer synchronous module loading and simplicity.
Use ES Modules when:
- You're developing universal JavaScript applications that run both on the server and in the browser.
- You require asynchronous module loading to improve performance and non-blocking behavior.
Dynamic Imports and Their Use Cases
Dynamic imports introduce a way to load modules on demand using the import() function, which returns a promise. This feature is particularly useful in scenarios where you want to reduce the initial load time of your application by splitting the code and loading modules only when needed, commonly known as code splitting. Dynamic imports are supported in both CommonJS and ES Modules, enabling more flexible and efficient code organization.
Asynchronous Module Loading with async/await
Asynchronous module loading can be achieved using the async/await syntax with dynamic imports, allowing developers to load modules in a non-blocking manner. This approach is beneficial for improving application performance, especially in web applications where minimizing resource loading time is crucial. By leveraging async/await with dynamic imports, developers can ensure that modules are loaded exactly when needed, optimizing resource utilization and enhancing the user experience.
Module Design Patterns
In the realm of Node.js development, adopting a module design pattern is a strategic approach to structuring and organizing code. These patterns not only aid in creating a more readable and maintainable codebase but also in encapsulating functionality in a logical manner. This section explores various module design patterns, including the revealing module pattern and singleton pattern, and provides guidance on selecting the appropriate pattern based on your project's needs.
Exploring Different Patterns for Organizing and Structuring Modules
Revealing Module Pattern: This pattern involves defining all of your module's functionalities within the scope of a single function or object. Only the necessary parts are exposed to the outside world, while the rest of the module's internals remain private. This approach offers a clear distinction between the public interface and private implementation details, enhancing code clarity and maintainability.
Benefits:
The revealing module pattern provides a structured and intuitive way to organize modules, making it easier to understand the module's capabilities at a glance. It also protects the module's internal state and behavior from unintended interference.
Singleton Pattern:
The singleton pattern ensures that a class has only one instance and provides a global point of access to it. In Node.js, this pattern can be implemented by creating a module that exports a single instance of an object or class. This is particularly useful when you need a single object to coordinate actions across the system.
Choosing the Right Pattern Based on Your Project's Needs
Selecting the most suitable module design pattern depends on several factors related to your project's structure, functionality, and specific requirements. Consider the following criteria when choosing a module design pattern:
- Complexity and Size of the Module: For larger, more complex modules, the revealing module pattern can help organize functionalities clearly, making the code easier to navigate and manage. In contrast, simpler modules or those requiring a single point of access might benefit from the singleton pattern.
- Reusability and Encapsulation Needs: If your module contains functionalities that need to be reused across different parts of the application without creating new instances, the singleton pattern might be the right choice. However, if you need to encapsulate logic and expose only certain parts of the module, the revealing module pattern could be more appropriate.
- Maintainability and Scalability: Consider how the chosen pattern will affect the long-term maintainability and scalability of your code. Patterns that clearly separate concerns and encapsulate details are generally easier to maintain and extend.
Best Practices and Tips for Module Usage in Node.js Projects
In the dynamic and modular world of Node.js development, adopting best practices for module usage is crucial for building efficient, secure, and maintainable applications. This section outlines key recommendations, highlights common pitfalls to avoid, and offers guidance on testing, debugging, and keeping your modules up-to-date. By adhering to these practices, you can enhance the reliability and performance of your Node.js projects.
Recommendations for Effective Module Usage
- Modularize Thoughtfully: Organize your code into modules with clear, focused responsibilities. This not only improves readability and maintainability but also facilitates easier testing and debugging.
- Use Semantic Versioning: When managing dependencies, pay close attention to semantic versioning. Opt for fixed version numbers in your package.json to avoid unexpected updates that might break your application.
- Leverage NPM Scripts: Utilize npm scripts for common tasks such as testing, building, and deploying. This can streamline your development workflow and ensure consistency across environments.
Avoiding Common Pitfalls and Mistakes
- Over-Modularization: While modularization is beneficial, excessive fragmentation can lead to complexity and overhead. Aim for a balance that suits your project's scale and complexity.
- Ignoring Dependency Management: Regularly audit your project's dependencies for vulnerabilities and updates. Neglecting dependency management can expose your application to security risks and outdated packages.
- Circular Dependencies: Be vigilant about circular dependencies as they can lead to subtle and hard-to-debug issues. Design your module architecture to minimize interdependencies.
Testing and Debugging Modules for Reliability and Maintainability
- Write Unit Tests: Ensure each module has accompanying unit tests. This promotes reliability and makes refactoring safer and easier.
- Utilize Debugging Tools: Leverage Node.js's built-in debugger or external tools like node-inspect to troubleshoot issues in your modules. Consistent use of logging can also aid in monitoring and debugging.
- Embrace Test-Driven Development (TDD): Adopting TDD practices can help in designing more modular and testable code, thereby enhancing the overall quality of your modules.
Keeping Modules Up-to-Date with Security Patches and New Features
- Stay Informed About Vulnerabilities: Use tools like npm audit or Snyk to scan your projects for known vulnerabilities and apply updates or patches as necessary.
- Regularly Review Dependencies: Periodically review and update your project's dependencies to leverage new features and improvements, while ensuring compatibility.
- Deprecate Unused Dependencies: Remove or replace unused or deprecated dependencies to keep your project lean and reduce potential attack surfaces.
Conclusion
Node.js modules stand at the core of developing scalable, efficient, and organized applications. Throughout this guide, we've explored the intricacies of module creation, management, and best practices, from the basics of module systems to advanced design patterns and maintenance strategies. By understanding the significance of modules, leveraging npm for dependency management, adopting suitable module design patterns, and adhering to best practices for testing and security, developers can craft robust Node.js applications that stand the test of time.
Transform your application performance with leading
Node JS Development Service Companies.
share this page if you liked it 😊
Other Related Blogs
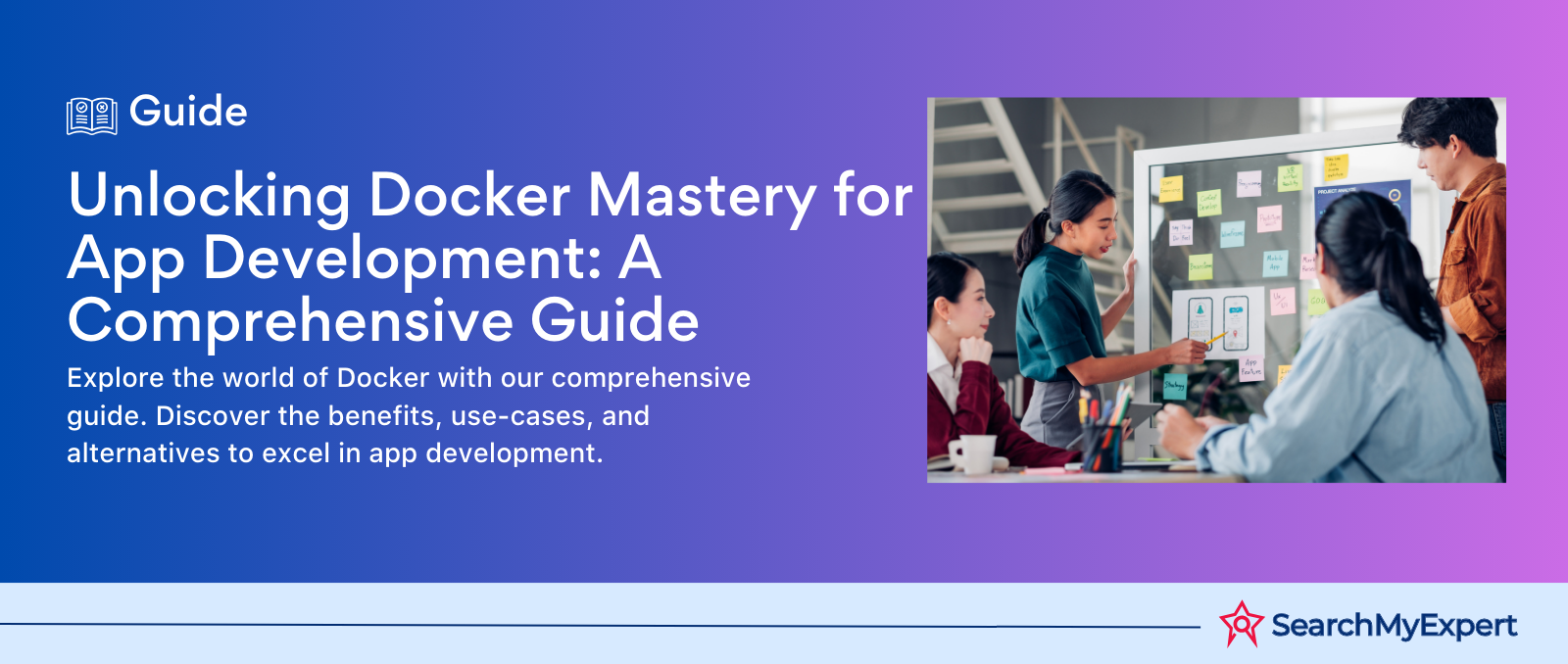
Mastering Docker for App Development: A Comprehensive Guide to Benefits, Use-Cases, and Alternatives
STAY UP TO DATE
GET PATH'S LATEST
Receive bi-weekly updates from the SME, and get a heads up on upcoming events.
Contact Us
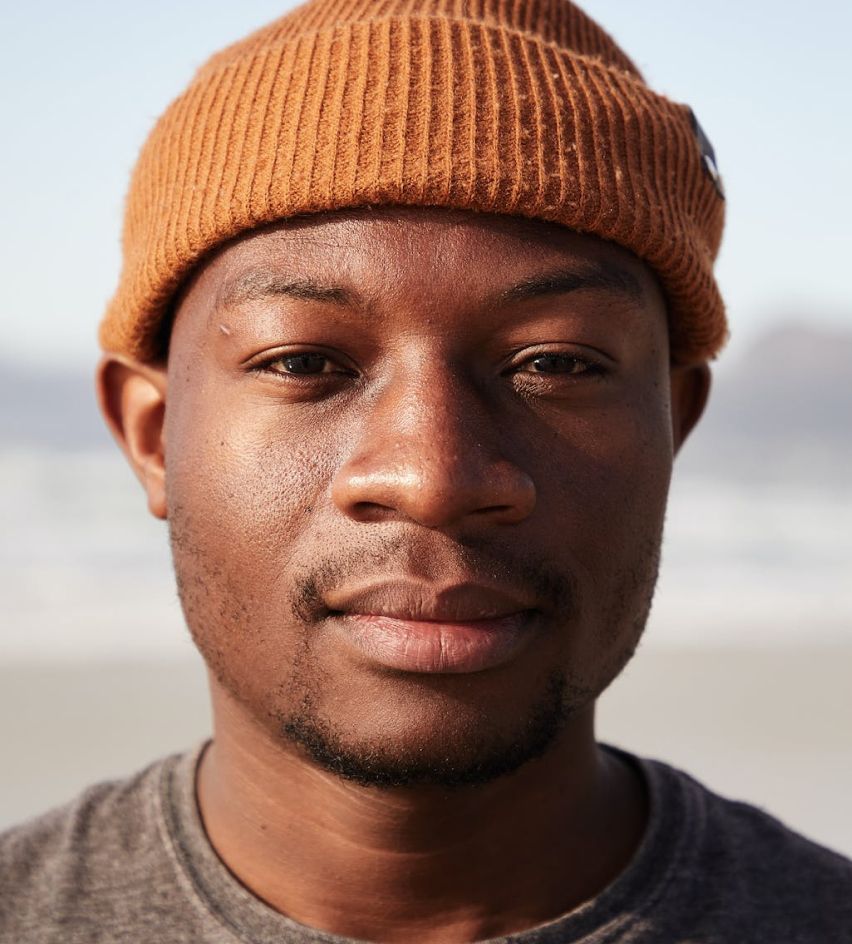
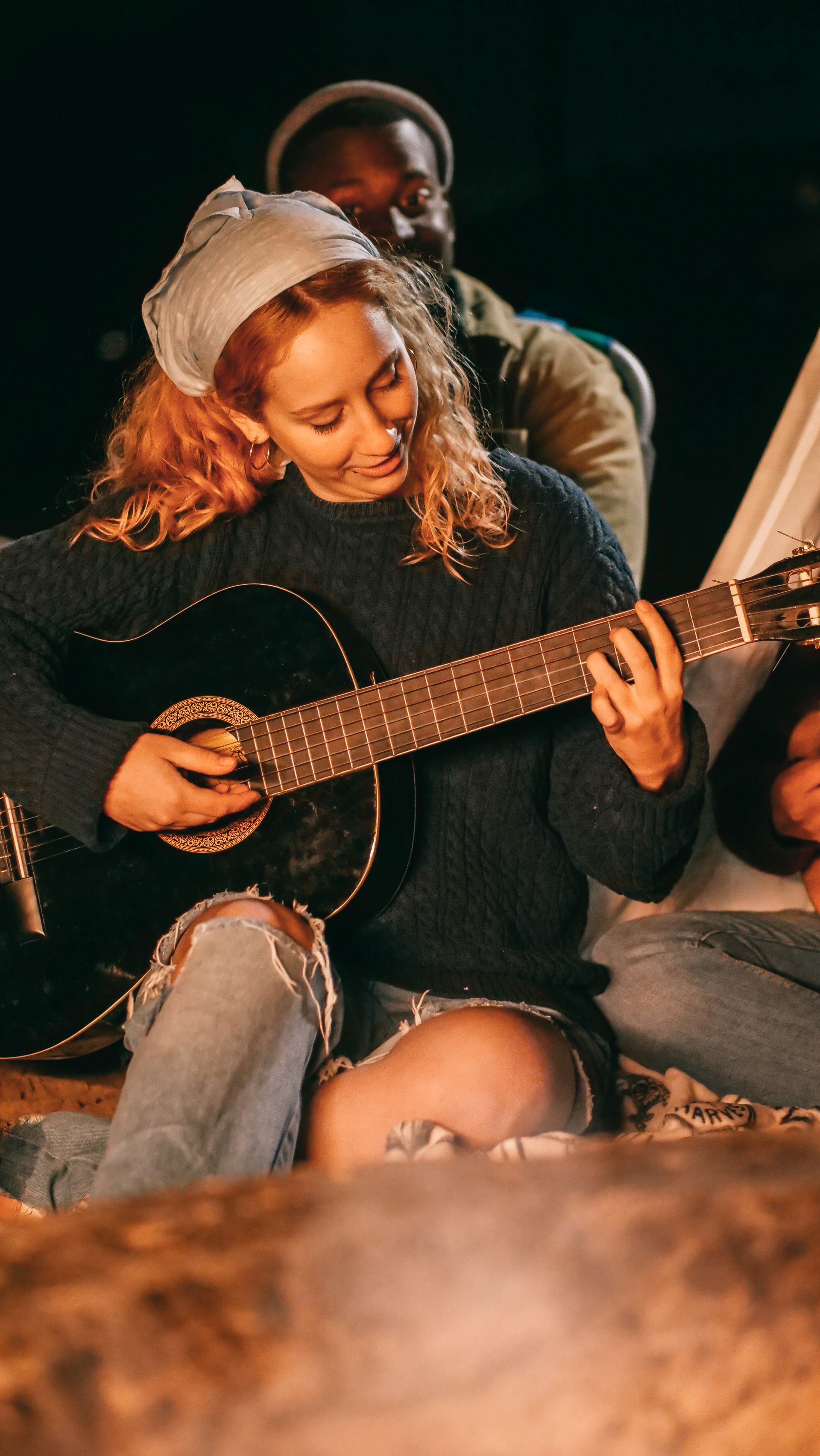